Python function to filter numbers by threshold
Python Filter: Exercise-3 with Solution
Write a Python function that filters out all elements less than or equal than a specified value from a list of numbers using the filter function.
Sample Solution:
Python Code:
def filter_numbers_less_than(numbers, threshold):
"""
Filters out all elements less than or equal to a specified value from a list of numbers.
Args:
numbers (list): A list of numbers.
threshold (float): The threshold value to compare against.
Returns:
list: A new list containing only the numbers less than or equal to the threshold.
"""
# Define the filtering function
def is_less_than_threshold(num):
return num <= threshold
# Use the filter function to filter out numbers
filtered_numbers = list(filter(is_less_than_threshold, numbers))
return filtered_numbers
# Example usage:
numbers = [20, 15, 24, 37, 23, 11, 7]
print("Original list of numbers:",numbers)
threshold = 20
print("\nFilters out all elements less than or equal to a specified value",threshold,":")
result = filter_numbers_less_than(numbers, threshold)
print(result)
Explanation:
In the exercise above -
- The "filter_numbers_less_than()" function takes two arguments: numbers (a list of numbers) and a threshold (the value to compare against).
- Inside the function, we define a nested function "is_less_than_threshold()" that checks if a number is greater than or equal to the specified threshold.
- We use the filter function to filter out numbers from the numbers list by applying the "is_less_than_threshold()" function as the filtering condition.
- The filtered numbers are converted to a list and returned as the result.
Sample Output:
Original list of numbers: [20, 15, 24, 37, 23, 11, 7] Filters out all elements less than or equal to a specified value 20 : [20, 15, 11, 7]
Flowchart:
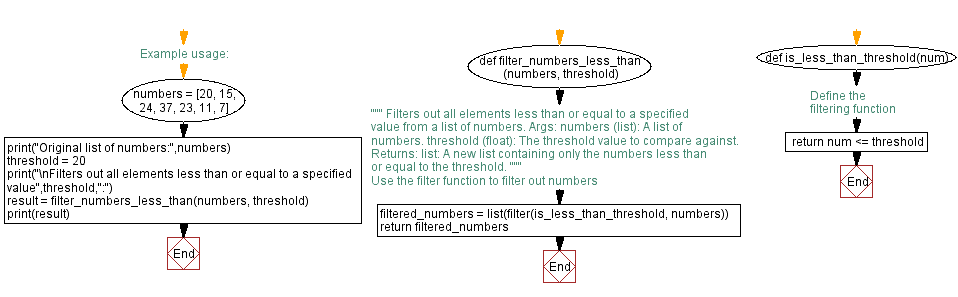
Python Code Editor:
Previous: Python program to extract uppercase letters.
Next: Python program to extract names starting with vowels.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/filter/python-filter-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics