Python program to extract names starting with vowels
Write a Python program that creates a list of names and uses the filter function to extract names that start with a vowel (A, E, I, O, U).
Sample Solution:
Python Code:
# Define a list of names
names = ["Elita", "Vitold", "Audovacar", "Kerensa", "Ramana", "Iolanda", "Landyn"]
print("Original list of names:")
print(names)
# Define a function to check if a name starts with a vowel
def starts_with_vowel(name):
return name[0].lower() in ['a', 'e', 'i', 'o', 'u']
# Use the filter function to extract names starting with a vowel
vowel_names = list(filter(starts_with_vowel, names))
print("\nExtract names starting with a vowel:")
# Print the extracted names
print(vowel_names)
Explanation:
In the exercise above -
- Start with a list of names called "names".
- Define a function called "starts_with_vowel()" that checks if the first letter of a name (converted to lowercase) is in the list of vowels.
- Next we use the filter function to filter the names from the names list based on the conditions defined by the "starts_with_vowel()" function.
- The filtered names are converted to a list, and we print the extracted names.
Sample Output:
Original list of names: ['Elita', 'Vitold', 'Audovacar', 'Kerensa', 'Ramana', 'Iolanda', 'Landyn'] Extract names starting with a vowel: ['Elita', 'Audovacar', 'Iolanda']
Flowchart:
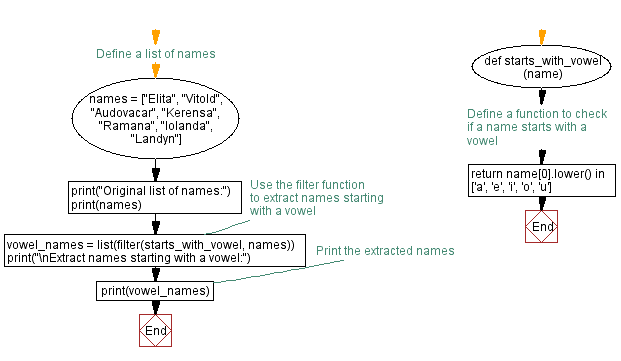
Python Code Editor:
Previous: Python function to filter numbers by threshold.
Next: Python function to filter out empty strings from a list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics