Python program to filter prime numbers
Write a Python program that filters out prime numbers from a list of integers using the filter function.
Sample Solution:
Python Code:
# Define a function to check if a number is prime
def is_prime(n):
if n <= 1:
return False
if n <= 3:
return True
if n % 2 == 0 or n % 3 == 0:
return False
i = 5
while i * i <= n:
if n % i == 0 or n % (i + 2) == 0:
return False
i += 6
return True
# Define a list of integers
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17]
print("List of integers:")
print(numbers)
# Use the filter function to extract prime numbers
prime_numbers = list(filter(is_prime, numbers))
print("\nExtract prime numbers of the said list:")
# Print the extracted prime numbers
print(prime_numbers)
Explanation:
In the exercise above,
- First, we define a function called "is_prime()" that checks if a given number n is prime. This function uses the primality test with optimizations.
- Define a list called 'numbers' containing integers.
- Next, we use the filter function to filter out prime numbers from the numbers list by applying the "is_prime()" function as the filtering condition.
- The filtered prime numbers are converted to a list, and we print the extracted prime numbers.
Sample Output:
List of integers: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17] Extract prime numbers of the said list: [2, 3, 5, 7, 11, 13, 17]
Flowchart:
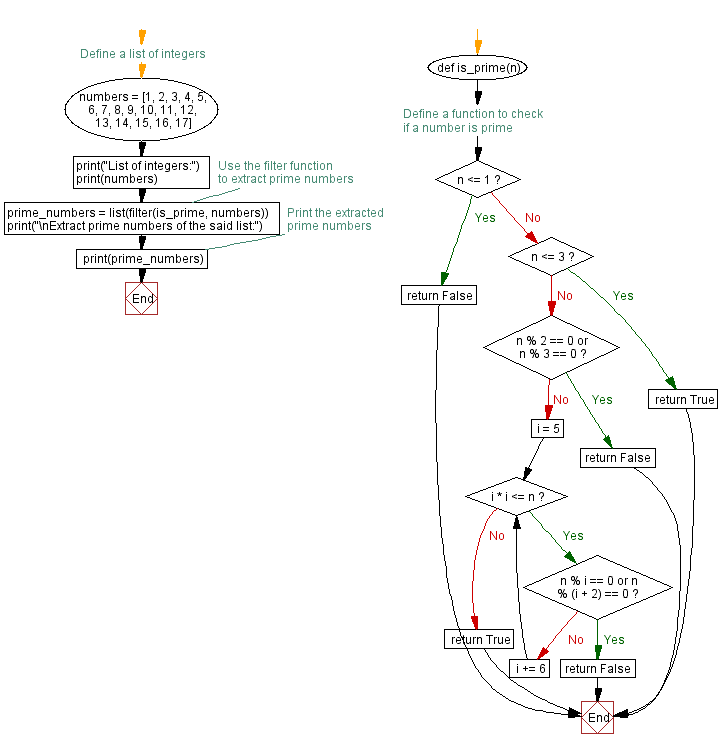
Python Code Editor:
Previous: Python program to filter students by high grades.
Next: Python program to extract words with more than five letters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics