Python program to extract words with more than five letters
Python Filter: Exercise-8 with Solution
Write a Python program that creates a list of words and use the filter function to extract words that contain more than five letters.
Sample Solution:
Python Code:
# Define a list of words
words = ["Red", "Green", "Orange", "White", "Black", "Pink", "Yellow"]
print("List of words:")
print(words)
# Define a function to check if a word has more than five letters
def has_more_than_five_letters(word):
return len(word) > 5
# Use the filter function to extract words with more than five letters
long_words = list(filter(has_more_than_five_letters, words))
print("\nExtract words with more than five letters:")
# Print the extracted long words
print(long_words)
Explanation:
In the exercise above -
- First, we define a list called 'words' containing words as strings.
- Define a function called "has_more_than_five_letters()" that checks if a word has more than five letters by comparing its length to 5.
- Use the filter function to filter out words from the words list based on the condition defined by the "has_more_than_five_letters()" function.
- Finally, the filtered words with more than five letters are converted to a list, and we print the extracted long words.
Sample Output:
List of words: ['Red', 'Green', 'Orange', 'White', 'Black', 'Pink', 'Yellow'] Extract words with more than five letters: ['Orange', 'Yellow']
Flowchart:
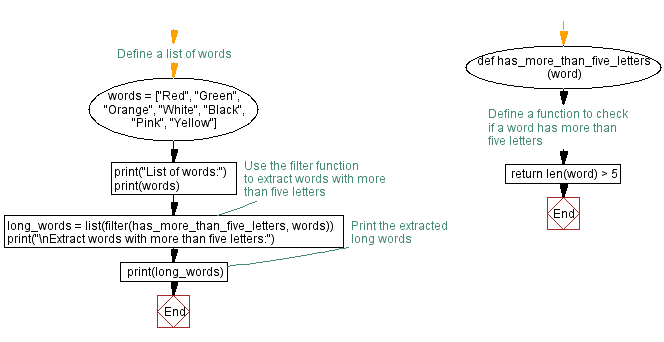
Python Code Editor:
Previous: Python program to filter prime numbers.
Next: Python function to filter strings with a specific substring.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/filter/python-filter-exercise-8.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics