Python Generators: Yielding cubes from 1 to n
Python: Generators Yield Exercise-1 with Solution
Write a Python program that creates a generator function that yields cubes of numbers from 1 to n. Accept n from the user.
Sample Solution:
Python Code:
def cube_generator(n):
for i in range(1, n + 1):
yield i ** 3
# Accept input from the user
n = int(input("Input a number: "))
# Create the generator object
cubes = cube_generator(n)
# Iterate over the generator and print the cubes
print("Cubes of numbers from 1 to", n)
for num in cubes:
print(num)
Sample Output:
Input a number: 10 Cubes of numbers from 1 to 10 1 8 27 64 125 216 343 512 729 1000
Explanation:
In the above exercise -
- Inside the "cube_generator()" function, a for loop iterates over the range of numbers from 1 to n + 1. For each number i, it calculates the cube by raising i to the power of 3 using the ** operator.
- Instead of using the return statement to return the cube value, it uses the yield keyword. This makes the function a generator, allowing it to generate and yield one cube value at a time when requested.
- The program prompts the user to input a number using the input() function. The input value is then converted to an integer using int() and stored in n.
- The generator object "cubes" is created by calling the cube_generator function with n. This sets up the generator and prepares it for iteration.
- The program then iterates over the "cubes" generator using a for loop. For each iteration, the next cube value is generated and assigned to the variable num.
- The cube values are printed one by one inside the loop using print().
Flowchart:
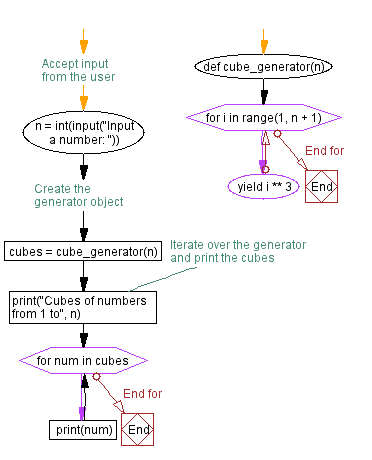
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Python Generators Yield Exercise Home.
Next: Python random number generator using generators.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/generators-yield/python-generators-yield-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics