Python prime number generator using generators
10. Prime Generator (Duplicate Variation)
Write a Python program that creates a generator function that generates all prime numbers between two given numbers.
A prime number (or a prime) is a natural number greater than 1 that is not a product of two smaller natural numbersFor example, 5 is prime because the only ways of writing it as a product, 1 × 5 or 5 × 1, involve 5 itself.
Sample Solution:
Python Code:
def is_prime_num(n):
# Check if the number is prime
if n < 2:
return False
for i in range(2, int(n ** 0.5) + 1):
if n % i == 0:
return False
return True
def prime_numbers(start, end):
# Generate prime numbers between start and end
for num in range(start, end + 1):
if is_prime_num(num):
yield num
# Accept input from the user
start = int(input("Input the starting number: "))
end = int(input("Input the ending number: "))
# Create the prime numbers generator
prime_gen = prime_numbers(start, end)
# Generate and print all prime numbers
print("Prime numbers between", start, "and", end, "are:")
for prime in prime_gen:
print(prime, end=",")
Sample Output:
Input the starting number: 1 Input the ending number: 30 Prime numbers between 1 and 30 are: 2,3,5,7,11,13,17,19,23,29,
Input the starting number: 100 Input the ending number: 200 Prime numbers between 100 and 200 are: 101,103,107,109,113,127,131,137,139,149,151,157,163,167,173,179,181,191,193,197,199,
Explanation:
In the above exercise,
- The "is_prime_num()" function is used to check whether a number is prime. It iterates from 2 to the square root of the number and checks for any divisors.
- The prime_numbers generator function generates prime numbers between the given start and end numbers. It iterates from start to end and checks each number whether it is prime using the is_prime function. If it is prime, it yields the number.
- The program accepts starting and ending numbers from the user. It then creates the prime_numbers generator using these input values. Finally, it iterates over the generator and prints all the prime numbers in the specified range.
Flowchart:
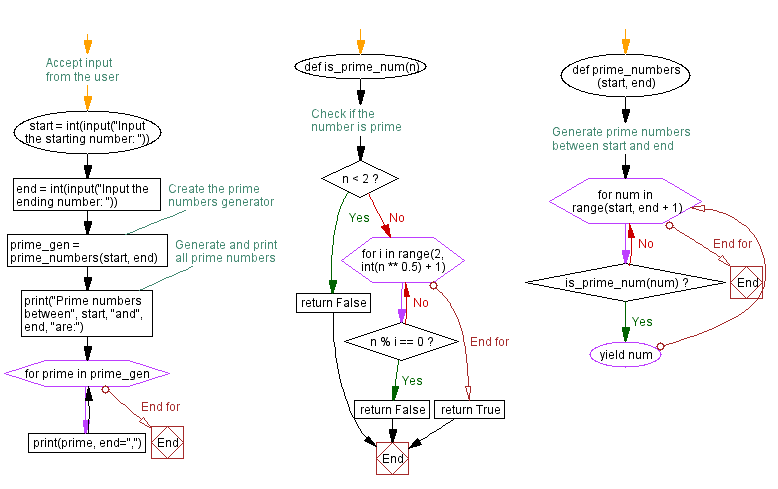
For more Practice: Solve these Related Problems:
- Write a Python program to create a generator that yields all prime numbers between two limits and then count the total primes generated.
- Write a Python function that yields prime numbers in a specified range and prints each prime alongside its sequence index.
- Write a Python script to stop the prime generator once a prime exceeding a given threshold is found, and then print that prime.
- Write a Python program to yield prime numbers and filter out only those that are palindromic, printing the filtered list.
Go to:
Previous: Python prime factors generator using generators.
Next: Generate all string permutations.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.