Python permutations generator: Generate all string permutations
11. String Permutations Generator
Write a Python program to create a generator that generates all possible permutations of a string.
Sample Solution:
Python Code:
def string_permutations(string):
if len(string) <= 1:
yield string
else:
for i in range(len(string)):
current_char = string[i]
remaining_chars = string[:i] + string[i+1:]
for permutation in string_permutations(remaining_chars):
yield current_char + permutation
# Accept input from the user
input_string = input("Input a string: ")
# Create the string permutations generator
permutations_gen = string_permutations(input_string)
# Generate and print all permutations
print("All permutations of", input_string + ":")
for permutation in permutations_gen:
print(permutation, end = ", ")
Sample Output:
Input a string: abc All permutations of abc: abc, acb, bac, bca, cab, cba,
Input a string: print All permutations of print: print, pritn, prnit, prnti, prtin, prtni, pirnt, pirtn, pinrt, pintr, pitrn, pitnr, pnrit, pnrti, pnirt, pnitr, pntri, pntir, ptrin, ptrni, ptirn, ptinr, ptnri, ptnir, rpint, rpitn, rpnit, rpnti, rptin, rptni, ripnt, riptn, rinpt, rintp, ritpn, ritnp, rnpit, rnpti, rnipt, rnitp, rntpi, rntip, rtpin, rtpni, rtipn, rtinp, rtnpi, rtnip, iprnt, iprtn, ipnrt, ipntr, iptrn, iptnr, irpnt, irptn, irnpt, irntp, irtpn, irtnp, inprt, inptr, inrpt, inrtp, intpr, intrp, itprn, itpnr, itrpn, itrnp, itnpr, itnrp, nprit, nprti, npirt, npitr, nptri, nptir, nrpit, nrpti, nript, nritp, nrtpi, nrtip, niprt, niptr, nirpt, nirtp, nitpr, nitrp, ntpri, ntpir, ntrpi, ntrip, ntipr, ntirp, tprin, tprni, tpirn, tpinr, tpnri, tpnir, trpin, trpni, tripn, trinp, trnpi, trnip, tiprn, tipnr, tirpn, tirnp, tinpr, tinrp, tnpri, tnpir, tnrpi, tnrip, tnipr, tnirp,
Explanation:
In the above exercise,
- The string_permutations generator function generates all possible permutations of a given string.
- It uses recursive logic to generate permutations.
- If the string length is less than or equal to 1, it yields the string itself. Otherwise, it iterates over each character in the string.
- For each character, it generates all permutations of the remaining characters by calling the string_permutations function recursively.
- It yields the current character concatenated with each permutation.
- Next the program accepts a string from the user. It then creates the string_permutations generator using the input string.
Finally, it iterates over the generator and prints all the permutations of the input string.
Flowchart:
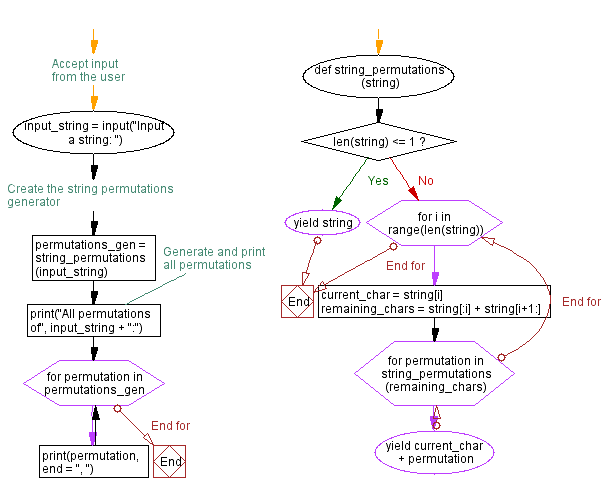
For more Practice: Solve these Related Problems:
- Write a Python program to create a generator that yields all possible permutations of a given string, including duplicates.
- Write a Python function that uses a generator to yield permutations of a string and then prints only those permutations that have a specific length.
- Write a Python script to generate all permutations of a string and count the total number of permutations, printing the count.
- Write a Python program to yield string permutations in lexicographical order and then print them one per line.
Go to:
Previous: Python prime number generator using generators.
Next: Generate the next happy number.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.