Python square root generator: Generate square, cube roots of numbers
13. Square and Cube Roots Generator
Write a Python program to create a generator that generates the square, cube roots of numbers from 1 to n.
Sample Solution:
Python Code:
import math
def roots_generator(n):
for i in range(1, n+1):
yield math.sqrt(i), i**(1/3)
# Accept input from the user
n = int(input("Input a number: "))
# Create the roots generator
roots_gen = roots_generator(n)
# Generate and print the square roots and cube roots
print("Square roots and cube roots of numbers from 1 to", n)
for i, (square_root, cube_root) in enumerate(roots_gen, start=1):
print("Number:", i)
print("Square root:", square_root)
print("Cube root:", cube_root)
print()
Sample Output:
Input a number: 10 Square roots and cube roots of numbers from 1 to 10 Number: 1 Square root: 1.0 Cube root: 1.0 Number: 2 Square root: 1.4142135623730951 Cube root: 1.2599210498948732 Number: 3 Square root: 1.7320508075688772 Cube root: 1.4422495703074083 Number: 4 Square root: 2.0 Cube root: 1.5874010519681994 Number: 5 Square root: 2.23606797749979 Cube root: 1.7099759466766968 Number: 6 Square root: 2.449489742783178 Cube root: 1.8171205928321397 Number: 7 Square root: 2.6457513110645907 Cube root: 1.912931182772389 Number: 8 Square root: 2.8284271247461903 Cube root: 2.0 Number: 9 Square root: 3.0 Cube root: 2.080083823051904 Number: 10 Square root: 3.1622776601683795 Cube root: 2.154434690031884
Explanation:
In the above exercise,
- The "roots_generator()" function is a generator function that yields the square root and cube root of numbers from 1 to n. It uses the math.sqrt() function from the math module to calculate the square root and the exponentiation operator ** to calculate the cube root.
- The program accepts a number from the user as n. It then creates the roots_generator generator from the input number. Finally, the enumerate function is used to iterate over the roots_gen generator and obtain both the index i and the square root and cube root values. By using enumerate with the start=1 parameter, the index i starts from 1. This allows us to print the correct number associated with each pair of roots.
Flowchart:
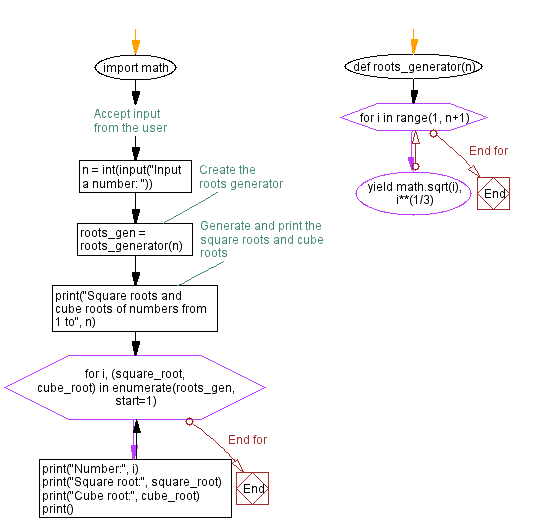
For more Practice: Solve these Related Problems:
- Write a Python program to create a generator that yields a tuple containing the square root and cube root of each number from 1 to n.
- Write a Python function that generates and prints both the square root and cube root for numbers 1 to n, formatted to two decimal places.
- Write a Python script to compute the sum of square roots and cube roots for numbers in a range using a generator, then print the total.
- Write a Python program to iterate over a generator that yields (sqrt, cbrt) tuples and then print the values in a tabulated format.
Go to:
Previous: Generate the next happy number.
Next: Generate next Armstrong numbers.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.