Python Running Average Generator: Calculate the average of a sequence
Python: Generators Yield Exercise-16 with Solution
Write a Python program to implement a generator function that generates the running average of a sequence of numbers.
Sample Solution:
Python Code:
def running_average_generator():
total = 0
count = 0
while True:
value = yield total / count if count > 0 else 0
total += value
count += 1
# Create the generator object
running_avg_gen = running_average_generator()
# Prime the generator
next(running_avg_gen)
# Accept input from the user
print("Input a sequence of numbers (Press Enter & 'e' to quit):")
while True:
num = input("> ")
if num.lower() == 'e':
break
value = float(num)
average = running_avg_gen.send(value)
print("Running Average:", average)
Sample Output:
Input a sequence of numbers (Press Enter & 'e' to quit): > 5 Running Average: 5.0 > 6 Running Average: 5.5 > 7 Running Average: 6.0 > e
Input a sequence of numbers (Press Enter & 'e' to quit): > -1 Running Average: -1.0 > -2 Running Average: -1.5 > -3 Running Average: -2.0 > -4 Running Average: -2.5 > e
Explanation:
In the above exercise,
The generator function running_average_generator() maintains a running total (total) and count (count) of the numbers received. It continuously yields the running average by dividing the total by the count (if count > 0) or 0 if count is 0.
We create the generator object running_avg_gen and prime it by calling next(running_avg_gen) before entering the input loop. We accept a sequence of numbers from the user until they enter 'e' to quit. Each number is sent to the generator using the send() method, and the running average is printed.
Flowchart:
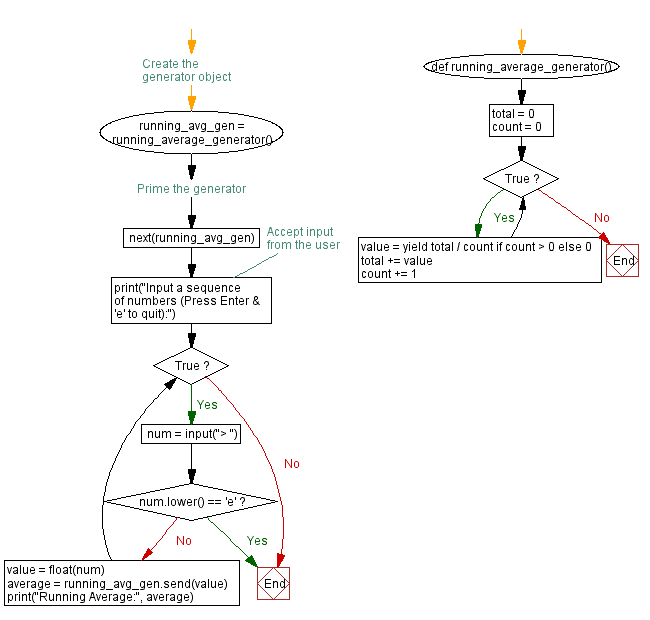
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Generate factors for a number.
Next: Generate the powers of a number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/generators-yield/python-generators-yield-exercise-16.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics