Python powers generator: Generate the powers of a number
17. Powers Generator
Write a Python program to create a generator function that generates the powers of a number up to a specified exponent.
Sample Solution:
Python Code:
def power_generator(base, exponent):
result = 1
for i in range(exponent + 1):
yield result
result *= base
# Accept input from the user
base = int(input("Input the base number: "))
exponent = int(input("Input the exponent: "))
# Create the generator object
power_gen = power_generator(base, exponent)
# Generate and print the powers
print(f"Powers of {base} up to exponent {exponent}:")
for power in power_gen:
print(power)
Sample Output:
Enter the base number: 2 Enter the exponent: 3 Powers of 2 up to exponent 3: 1 2 4 8
Input the base number: 8 Input the exponent: 4 Powers of 8 up to exponent 4: 1 8 64 512 4096
Explanation:
The generator function power_generator() takes a base number and an exponent as parameters. It uses a loop to calculate the powers of the base number, starting from 0 up to the specified exponent. The current power is yielded at each iteration.
We accept user input for the base number and exponent. Then, we create the generator object power_gen by calling the power_generator() function with the provided base and exponent.
Finally, we iterate over the generator and print each power of the base number up to the specified exponent.
Flowchart:
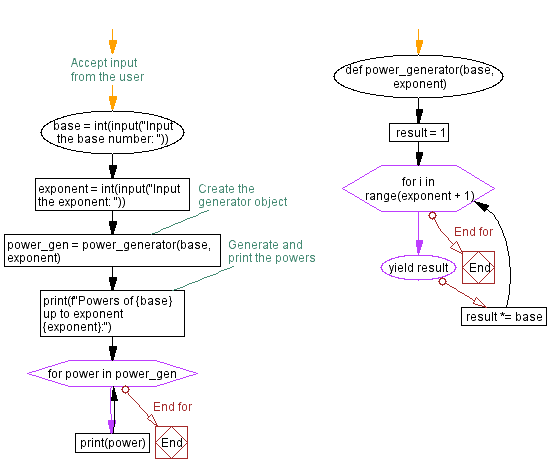
For more Practice: Solve these Related Problems:
- Write a Python program to create a generator that yields powers of a given base from exponent 0 up to n, and then prints each power.
- Write a Python function that accepts a base and maximum exponent, uses a generator to calculate the powers, and returns a list of the resulting values.
- Write a Python script to generate powers of a number using a generator and then sum these powers, printing the total sum.
- Write a Python program to create a generator that yields powers of a number, then filter out only even powers and print them.
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Calculate the average of a sequence.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.