Python Collatz sequence generator using generators
7. Collatz Sequence Generator
Write a Python program to implement a generator that generates the Collatz sequence for a given number.
The Collatz sequence, also known as the Collatz conjecture or 3n+1 problem, is a sequence of numbers defined by the following rules:
- Start with any positive integer n.
- If n is even, divide it by 2 to get n/2.
- If n is odd, multiply it by 3 and add 1 to get 3n+1.
- Repeat the process, using the resulting number, until you reach the number 1.
For instance, starting with n = 12 and applying the function f without "shortcut", one gets the sequence 12, 6, 3, 10, 5, 16, 8, 4, 2, 1.
The number n = 19 takes longer to reach 1: 19, 58, 29, 88, 44, 22, 11, 34, 17, 52, 26, 13, 40, 20, 10, 5, 16, 8, 4, 2, 1.
Sample Solution:
Python Code:
def collatz_sequence(n):
while n != 1:
yield n
if n % 2 == 0:
n = n // 2
else:
n = 3 * n + 1
yield 1
# Accept input from the user
n = int(input("Input a positive integer (n): "))
# Generate and print the Collatz sequence
print("Collatz sequence:")
for num in collatz_sequence(n):
print(num, end=", ")
Sample Output:
Input a positive integer (n): 12 Collatz sequence: 12, 6, 3, 10, 5, 16, 8, 4, 2, 1,
Input a positive integer (n): 19 Collatz sequence: 19, 58, 29, 88, 44, 22, 11, 34, 17, 52, 26, 13, 40, 20, 10, 5, 16, 8, 4, 2, 1,
Explanation:
In the above exercise,
The "collatz_sequence()" function is a generator that generates the Collatz sequence for a given number n. It starts with n and repeatedly applies the Collatz rules until it reaches 1. Each number in the sequence is yielded using the yield keyword.
The program accepts a positive integer from the user and calls the collatz_sequence generator with that number. It iterates over the generated sequence and prints each number.
Flowchart:
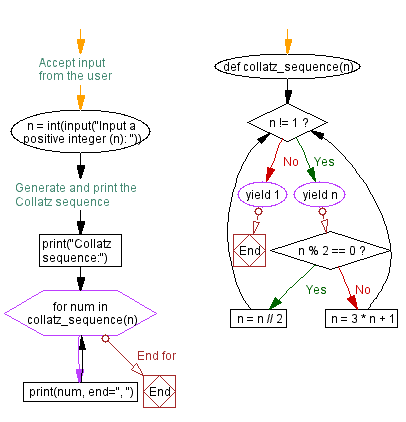
For more Practice: Solve these Related Problems:
- Write a Python program to implement a generator that yields the Collatz sequence starting from a user-specified number until it reaches 1.
- Write a Python function that generates the Collatz sequence for a given number and returns the number of steps taken to reach 1.
- Write a Python script to produce the Collatz sequence for several starting values and then print the sequences side by side.
- Write a Python program to generate the Collatz sequence for a given number and then compute the maximum value reached in the sequence.
Go to:
Previous: Python combinations generator using generators.
Next: Python next palindrome number generator using generators.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.