Python List Advanced Exercise - Reverse a list at a specific location
Python List Advanced: Exercise-1 with Solution
Write a Python function to reverse a list at a specific location.
Sample Solution:
Python Code:
# Define a function to reverse a portion of a list in place
def reverse_list_in_location(lst, start_pos, end_pos):
# Use a while loop to swap elements from the start and end positions
while start_pos < end_pos:
# Swap elements at start_pos and end_pos using tuple unpacking
lst[start_pos], lst[end_pos] = lst[end_pos], lst[start_pos]
# Move the start_pos towards the end_pos and vice versa
start_pos += 1
end_pos -= 1
# Return the modified list
return lst
# Create a list of numbers
nums = [10, 20, 30, 40, 50, 60, 70, 80]
# Define the start and end positions for the first reverse operation
start_pos = 2
end_pos = 4
# Print the original list
print("Original list:")
print(nums)
# Print a message indicating which portion of the list will be reversed
print("Reverse elements of the said list between index position " + str(start_pos) + " and " + str(end_pos))
# Call the reverse_list_in_location function and print the result
print(reverse_list_in_location(nums, start_pos, end_pos))
# Define the start and end positions for the second reverse operation
start_pos = 6
end_pos = 7
# Print a message indicating which portion of the list will be reversed
print("\nOriginal list:")
print(nums)
print("Reverse elements of the said list between index position " + str(start_pos) + " and " + str(end_pos))
# Call the reverse_list_in_location function again and print the result
print(reverse_list_in_location(nums, start_pos, end_pos))
# Define the start and end positions for the third reverse operation
start_pos = 0
end_pos = 7
# Print a message indicating which portion of the list will be reversed
print("\nOriginal list:")
print(nums)
print("Reverse elements of the said list between index position " + str(start_pos) + " and " + str(end_pos))
# Call the reverse_list_in_location function once more and print the result
print(reverse_list_in_location(nums, start_pos, end_pos))
Sample Output:
Original list: [10, 20, 30, 40, 50, 60, 70, 80] Reverse elements of the said list between index position 2 and 4 [10, 20, 50, 40, 30, 60, 70, 80] Original list: [10, 20, 50, 40, 30, 60, 70, 80] Reverse elements of the said list between index position 6 and 7 [10, 20, 50, 40, 30, 60, 80, 70] Original list: [10, 20, 50, 40, 30, 60, 80, 70] Reverse elements of the said list between index position 0 and 7 [70, 80, 60, 30, 40, 50, 20, 10]
Algorithm: reverse_list_in_location(lst, start_pos, end_pos) function
Input: A list “lst” and two integers start_pos and end_pos indicate the start and end positions of the sub-list that needs to be reversed.
Output: The input list “lst” with the sub-list from start_pos to end_pos reversed.
Steps:
- Initialize two variables start_pos and end_pos to the start and end positions of the sub-list.
- While start_pos is less than end_pos do the following:
- Swap the elements at start_pos and end_pos
- Increment start_pos by 1
- Decrement end_pos by 1
- Return the list lst.
Flowchart:
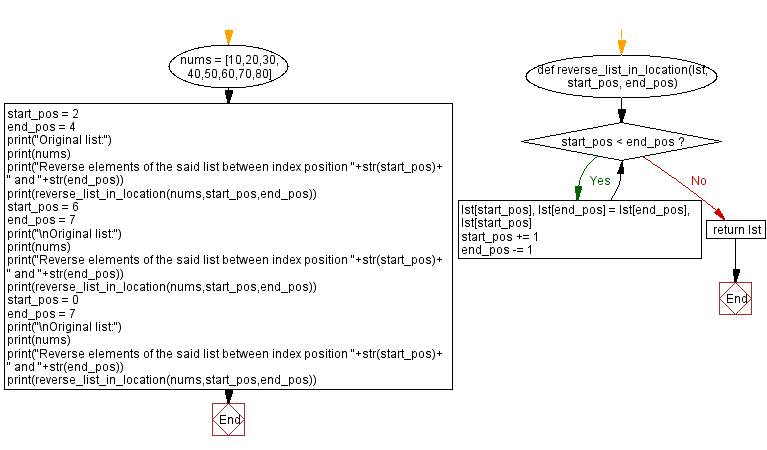
What is the time complexity and space complexity of the following Python code?
def reverse_list_in_location(lst, start_pos, end_pos):
while start_pos < end_pos:
lst[start_pos], lst[end_pos] = lst[end_pos], lst[start_pos]
start_pos += 1
end_pos -= 1
return lst
Time complexity - The time complexity of the code is O(n), where n is the difference between "end_pos" and "start_pos". Due to the swapping of two elements at each iteration, the while loop iterates n/2 times. Therefore, the time complexity of the while loop is O(n/2), which is equivalent to O(n).
Space complexity - The space complexity of the code is O(1) because the algorithm modifies the input list in-place without creating any new data structures. As a result, the function uses the same amount (constant) of space regardless of the size of the input list.
Python Code Editor:
Previous: Python List Advanced Exercise Home.
Next: Length of the longest increasing sub-sequence.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/list-advanced/python-list-advanced-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics