Python: Count integer in a given mixed list
Count Integers in Mixed List
Write a Python program to count integers in a given mixed list.
Sample Solution:
Python Code:
# Define a function 'count_integer' that counts the number of integers in a list
def count_integer(list1):
# Initialize a counter 'ctr' to 0
ctr = 0
# Iterate through the elements of 'list1'
for i in list1:
# Check if the current element is an integer using 'isinstance'
if isinstance(i, int):
# Increment the counter if the element is an integer
ctr = ctr + 1
# Return the count of integers in the list
return ctr
# Create a list 'list1' containing a mix of data types
list1 = [1, 'abcd', 3, 1.2, 4, 'xyz', 5, 'pqr', 7, -5, -12.22]
# Print a message indicating the original list
print("Original list:")
# Print the contents of 'list1'
print(list1)
# Print a message indicating that the number of integers in the list will be determined
print("\nNumber of integers in the said mixed list:")
# Call the 'count_integer' function with 'list1' and print the result
print(count_integer(list1))
Sample Output:
Original list: [1, 'abcd', 3, 1.2, 4, 'xyz', 5, 'pqr', 7, -5, -12.22] Number of integers in the said mixed list: 6
Flowchart:
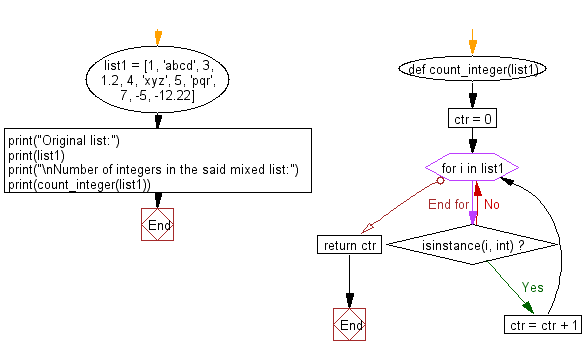
Python Code Editor:
Previous: Write a Python program to compute average of two given lists.
Next: Write a Python program to remove a specified column from a given nested list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics