Python: Calculate the product of the unique numbers of a given list
Python List: Exercise - 125 with Solution
Write a Python program to calculate the product of the unique numbers in a given list.
Sample Solution:
Python Code:
# Define a function 'unique_product' that calculates the product of unique elements in a list
def unique_product(list_data):
# Create a set 'temp' to store unique elements in the list
temp = list(set(list_data))
# Initialize a variable 'p' to store the product and set it to 1
p = 1
# Iterate through unique elements in 'temp'
for i in temp:
# Multiply the current element 'i' with 'p'
p *= i
# Return the product of unique elements
return p
# Create a list 'nums' containing integers with some duplicates
nums = [10, 20, 30, 40, 20, 50, 60, 40]
# Print a message indicating the original list
print("Original List:", nums)
# Print a message indicating the product of unique numbers
print("Product of the unique numbers of the said list:", unique_product(nums))
Sample Output:
Original List : [10, 20, 30, 40, 20, 50, 60, 40] Product of the unique numbers of the said list: 720000000
Flowchart:
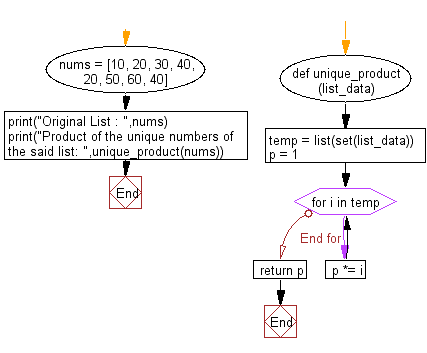
Python Code Editor:
Previous: Write a Python program to find the maximum and minimum product from the pairs of tuple within a given list.
Next: Write a Python program to interleave multiple lists of the same length.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/list/python-data-type-list-exercise-125.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics