Python: Interleave multiple lists of the same length
Python List: Exercise - 126 with Solution
Write a Python program to interleave multiple lists of the same length.
Sample Solution:
Python Code:
# Define a function 'interleave_multiple_lists' that interleaves elements from multiple lists
def interleave_multiple_lists(list1, list2, list3):
# Use a list comprehension with 'zip' to interleave elements from 'list1', 'list2', and 'list3'
result = [el for pair in zip(list1, list2, list3) for el in pair]
return result
# Create three lists 'list1', 'list2', and 'list3' with integer values
list1 = [1, 2, 3, 4, 5, 6, 7]
list2 = [10, 20, 30, 40, 50, 60, 70]
list3 = [100, 200, 300, 400, 500, 600, 700]
# Print a message indicating the original lists
print("Original list:")
print("list1:", list1)
print("list2:", list2)
print("list3:", list3)
# Print a message indicating the interleaved lists
print("\nInterleave multiple lists:")
# Call the 'interleave_multiple_lists' function with 'list1', 'list2', and 'list3' and print the result
print(interleave_multiple_lists(list1, list2, list3))
Sample Output:
Original list: list1: [1, 2, 3, 4, 5, 6, 7] list2: [10, 20, 30, 40, 50, 60, 70] list3: [100, 200, 300, 400, 500, 600, 700] Interleave multiple lists: [1, 10, 100, 2, 20, 200, 3, 30, 300, 4, 40, 400, 5, 50, 500, 6, 60, 600, 7, 70, 700]
Flowchart:
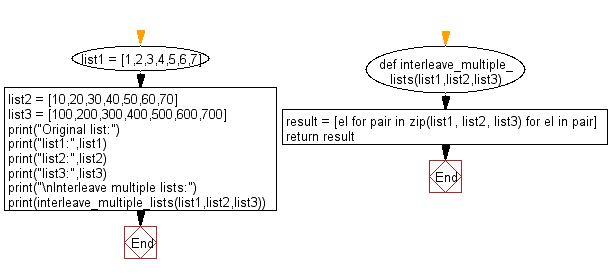
Python Code Editor:
Previous: Write a Python program to calculate the product of the unique numbers of a given list.
Next: Write a Python program to remove words from a given list of strings containing a character or string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/list/python-data-type-list-exercise-126.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics