Python: Sum of the numbers in a list between the indices of a specified range
Sum Numbers in List Within Index Range
Write a Python program to calculate the sum of the numbers in a list between the indices of a specified range.
Sample Solution:
Python Code:
# Define a function 'sum_Range_list' that calculates the sum of a specified range within a list
def sum_Range_list(nums, m, n):
# Initialize 'sum_range' to store the sum of the specified range
sum_range = 0
# Iterate through the list from index 'm' to 'n'
for i in range(m, n+1, 1):
# Add the value at the current index 'i' to 'sum_range'
sum_range += nums[i]
return sum_range
# Create a list of numbers 'nums'
nums = [2, 1, 5, 6, 8, 3, 4, 9, 10, 11, 8, 12]
# Print a message indicating the original list
print("Original list:")
print(nums)
# Set the range boundaries 'm' and 'n'
m = 8
n = 10
# Print a message indicating the specified range
print("Range:", m, ",", n)
# Print a message indicating the sum of the specified range
print("\nSum of the specified range:")
# Call the 'sum_Range_list' function with 'nums', 'm', and 'n', then print the result
print(sum_Range_list(nums, m, n))
Sample Output:
Original list: [2, 1, 5, 6, 8, 3, 4, 9, 10, 11, 8, 12] Range: 8 , 10 Sum of the specified range: 29
Flowchart:
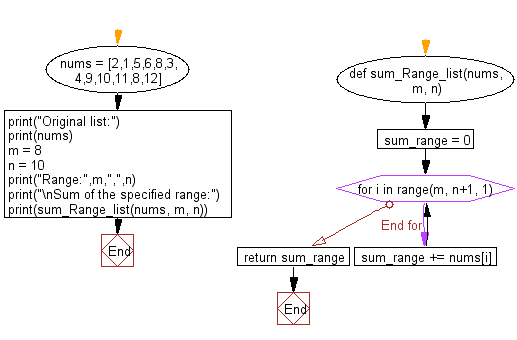
For more Practice: Solve these Related Problems:
- Write a Python program to calculate the sum of every nth element in a list.
- Write a Python program to sum all even-indexed elements in a list.
- Write a Python program to find the range of maximum sum subarrays.
- Write a Python program to compute rolling sums over a list.
Python Code Editor:
Previous: Write a Python program to remove words from a given list of strings containing a character or string.
Next: Write a Python program to reverse each list in a given list of lists.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics