Python: Reverse each list in a given list of lists
Reverse Each List in Nested Lists
Write a Python program to reverse each list in a given list of lists.
Sample Solution:
Python Code:
# Define a function 'reverse_list_lists' that reverses each list within a list of lists
def reverse_list_lists(nums):
# Iterate through each sublist 'l' in the 'nums' list
for l in nums:
# Sort each sublist 'l' in reverse order
l.sort(reverse=True)
return nums
# Create a list of lists 'nums'
nums = [[1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12], [13, 14, 15, 16]]
# Print a message indicating the original list of lists
print("Original list of lists:")
print(nums)
# Print a message indicating the operation to reverse each list
print("\nReverse each list in the said list of lists:")
# Call the 'reverse_list_lists' function with 'nums' and print the result
print(reverse_list_lists(nums))
Sample Output:
Original list of lists: [[1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12], [13, 14, 15, 16]] Reverse each list in the said list of lists: [[4, 3, 2, 1], [8, 7, 6, 5], [12, 11, 10, 9], [16, 15, 14, 13]]
Flowchart:
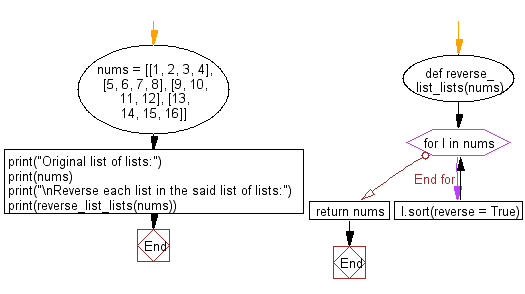
Python Code Editor:
Previous: Write a Python program to calculate the sum of the numbers in a list between the indices of a specified range.
Next: Write a Python program to count the same pair in three given lists.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics