Python: Find the difference between two list including duplicate elements
Difference Between Two Lists with Duplicates
Write a Python program to find the difference between two lists including duplicate elements.
Sample Solution:
Python Code:
# Define a function 'list_difference' that finds the difference between two lists (including duplicate elements)
def list_difference(l1, l2):
# Create a copy of 'l1' to avoid modifying the original list
result = list(l1)
# Iterate through elements in 'l2'
for el in l2:
# Remove the first occurrence of 'el' from 'result'
result.remove(el)
# Return the modified 'result' list containing the difference
return result
# Create two lists 'l1' and 'l2' with duplicate elements
l1 = [1, 1, 2, 3, 3, 4, 4, 5, 6, 7]
l2 = [1, 1, 2, 4, 5, 6]
# Print a message indicating the original lists
print("Original lists:")
# Print the contents of 'l1' and 'l2'
print(l1)
print(l2)
# Find the difference between 'l1' and 'l2' (including duplicate elements)
print("\nDifference between two said lists (including duplicate elements):")
# Call the 'list_difference' function with 'l1' and 'l2', then print the result
print(list_difference(l1, l2))
Sample Output:
Original lists: [1, 1, 2, 3, 3, 4, 4, 5, 6, 7] [1, 1, 2, 4, 5, 6] Difference between two said list including duplicate elements): [3, 3, 4, 7]
Flowchart:
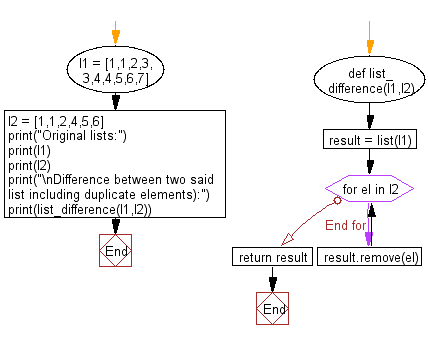
For more Practice: Solve these Related Problems:
- Write a Python program to find elements appearing only in one list.
- Write a Python program to calculate symmetric differences between lists.
- Write a Python program to remove duplicates before comparing lists.
- Write a Python program to count duplicate elements in two lists.
Go to:
Previous: Write a Python program to check common elements between two given list are in same order or not.
Next: Write a Python program to iterate over all pairs of consecutive items in a given list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.