Python: Sort a given list of strings(numbers) numerically
Sort List of String Numbers Numerically
Write a Python program to sort a given list of strings(numbers) numerically.
Sample Solution:
Python Code:
# Define a function 'sort_numeric_strings' that sorts a list of numeric strings numerically
def sort_numeric_strings(nums_str):
# Convert each numeric string to an integer and store the result in 'result'
result = [int(x) for x in nums_str]
# Sort the 'result' list in ascending order
result.sort()
return result
# Create a list of numeric strings 'nums_str'
nums_str = ['4', '12', '45', '7', '0', '100', '200', '-12', '-500']
# Print a message indicating the original list
print("Original list:")
# Print the contents of 'nums_str'
print(nums_str)
# Sort the list of numeric strings numerically using the 'sort_numeric_strings' function
print("\nSort the said list of strings (numbers) numerically:")
# Call the 'sort_numeric_strings' function with 'nums_str', then print the result
print(sort_numeric_strings(nums_str))
Sample Output:
Original list: ['4', '12', '45', '7', '0', '100', '200', '-12', '-500'] Sort the said list of strings(numbers) numerically: [-500, -12, 0, 4, 7, 12, 45, 100, 200]
Flowchart:
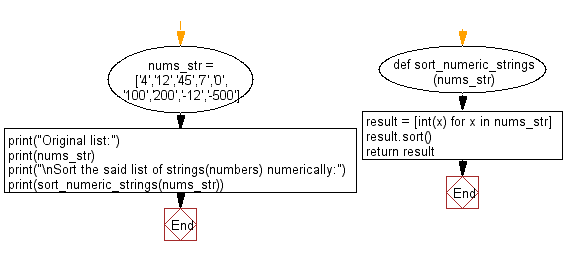
For more Practice: Solve these Related Problems:
- Write a Python program to sort a list of string numbers numerically while ignoring negative numbers.
- Write a Python program to sort a list of string numbers numerically but keep odd numbers before even numbers.
- Write a Python program to sort a list of string numbers numerically while treating ‘None’ values as zeros.
- Write a Python program to sort a list of string numbers numerically while maintaining their original string format.
Go to:
Previous: Write a Python program to sort a given mixed list of integers and strings. Numbers must be sorted before strings.
Next: Write a Python program to remove the specific item from a given list of lists.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.