Python: Generate a number in a specified range except some specific numbers
Python List: Exercise - 145 with Solution
Write a Python program to generate a number in a specified range except for some specific numbers.
Sample Solution:
Python Code:
# Import the 'choice' function from the 'random' module
from random import choice
# Define a function 'generate_random' that generates a random number within a specified range, excluding certain numbers
def generate_random(start_range, end_range, nums):
# Generate a random number within the specified range, excluding numbers in the 'nums' list
result = choice([i for i in range(start_range, end_range) if i not in nums])
return result
# Define the start and end range for the first random number generation
start_range = 1
end_range = 10
nums = [2, 9, 10]
# Print a message indicating the operation to generate a number within the specified range except for specific numbers
print("\nGenerate a number in a specified range (1, 10) except [2, 9, 10]")
# Call the 'generate_random' function with the specified range and excluded numbers, then print the result
print(generate_random(start_range, end_range, nums))
# Define the start and end range for the second random number generation
start_range = -5
end_range = 5
nums = [-5, 0, 4, 3, 2]
# Print a message indicating the operation to generate a number within the specified range except for specific numbers
print("\nGenerate a number in a specified range (-5, 5) except [-5, 0, 4, 3, 2]")
# Call the 'generate_random' function with the specified range and excluded numbers, then print the result
print(generate_random(start_range, end_range, nums))
Sample Output:
Generate a number in a specified range (1, 10) except [2, 9, 10] 7 Generate a number in a specified range (-5, 5) except [-5,0,4,3,2] -4
Flowchart:
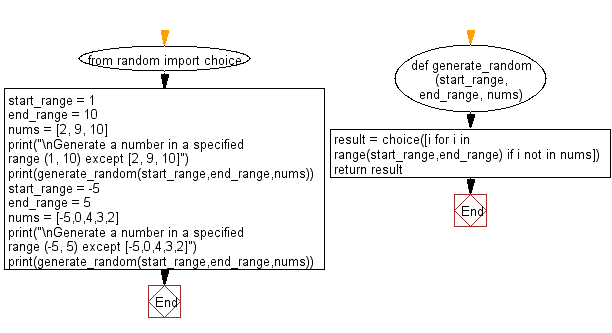
Python Code Editor:
Previous: Write a Python program to extract every first or specified element from a given two-dimensional list.
Next: Write a Python program to compute the sum of digits of each number of a given list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/list/python-data-type-list-exercise-145.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics