Python: Compute the sum of digits of each number of a given list
Sum of Digits in List Numbers
Write a Python program to compute the sum of digits of each number in a given list.
Sample Solution:
Python Code:
# Define a function 'sum_of_digits' that calculates the sum of digits in a list of integers
def sum_of_digits(nums):
# Use a nested generator expression to iterate over elements in 'nums'
# Convert each element to a string and iterate over its characters
# Use 'isdigit()' to check if a character is a digit, convert it to an integer, and sum them
return sum(int(el) for n in nums for el in str(n) if el.isdigit())
# Create a list of integers 'nums'
nums = [10, 2, 56]
# Print a message indicating the original list
print("Original tuple:")
print(nums)
# Print a message indicating the operation to calculate the sum of digits in the list
print("Sum of digits of each number of the said list of integers:")
# Call the 'sum_of_digits' function with 'nums' and print the result
print(sum_of_digits(nums))
# Create another list with a mix of integers and non-integer values
nums = [10, 20, 4, 5, 'b', 70, 'a']
# Print a message indicating the original list
print("\nOriginal tuple:")
print(nums)
# Print a message indicating the operation to calculate the sum of digits in the list
print("Sum of digits of each number of the said list of integers:")
# Call the 'sum_of_digits' function with 'nums' and print the result
print(sum_of_digits(nums))
# Create a list of integers, including negative values
nums = [10, 20, -4, 5, -70]
# Print a message indicating the original list
print("\nOriginal tuple:")
print(nums)
# Print a message indicating the operation to calculate the sum of digits in the list
print("Sum of digits of each number of the said list of integers:")
# Call the 'sum_of_digits' function with 'nums' and print the result
print(sum_of_digits(nums))
Sample Output:
Original tuple: [10, 2, 56] Sum of digits of each number of the said list of integers: 14 Original tuple: [10, 20, 4, 5, 'b', 70, 'a'] Sum of digits of each number of the said list of integers: 19 Original tuple: [10, 20, -4, 5, -70] Sum of digits of each number of the said list of integers: 19
Flowchart:
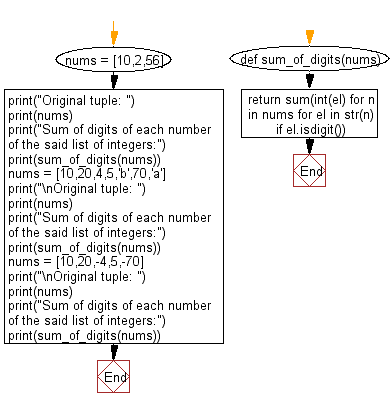
For more Practice: Solve these Related Problems:
- Write a Python program to compute the sum of the digits of numbers in a list, ignoring zeroes.
- Write a Python program to compute the sum of the digits of numbers in a list and return the smallest sum.
- Write a Python program to compute the sum of the digits of numbers in a list and count how many have an odd sum.
- Write a Python program to compute the sum of the digits of numbers in a list while treating negative numbers as positive.
Go to:
Previous: Write a Python program to generate a number in a specified range except some specific numbers.
Next: Write a Python program to interleave two given list into another list randomly.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.