Python: Join two given list of lists of same length, element wise
Join Two Lists of Lists Element-Wise
Write a Python program to join two given list of lists of the same length, element wise.
Sample Solution:
Python Code:
# Define a function called elementswise_join that takes two lists, 'l1' and 'l2', as input.
def elementswise_join(l1, l2):
# Use a list comprehension to element-wise join the elements of 'l1' and 'l2' using the 'zip' function.
result = [x + y for x, y in zip(l1, l2)]
# Return the result, which is the element-wise joined list.
return result
# Create two lists, 'nums1' and 'nums2', containing sublists of varying lengths.
nums1 = [[10, 20], [30, 40], [50, 60], [30, 20, 80]]
nums2 = [[61], [12, 14, 15], [12, 13, 19, 20], [12]]
# Print a message indicating the original lists.
print("Original lists:")
print(nums1)
print(nums2)
# Print a message indicating that the two lists are being element-wise joined, and call the 'elementswise_join' function.
print("\nJoin the said two lists element-wise:")
print(elementswise_join(nums1, nums2))
# Create two lists, 'list1' and 'list2', containing sublists of varying lengths.
list1 = [['a', 'b'], ['b', 'c', 'd'], ['e', 'f']]
list2 = [['p', 'q'], ['p', 's', 't'], ['u', 'v', 'w']]
# Print a message indicating the original lists.
print("\nOriginal lists:")
print(list1)
print(list2)
# Print a message indicating that the two lists are being element-wise joined, and call the 'elementswise_join' function.
print("\nJoin the said two lists element-wise:")
print(elementswise_join(list1, list2))
Sample Output:
Original lists: [[10, 20], [30, 40], [50, 60], [30, 20, 80]] [[61], [12, 14, 15], [12, 13, 19, 20], [12]] Join the said two lists element wise: [[10, 20, 61], [30, 40, 12, 14, 15], [50, 60, 12, 13, 19, 20], [30, 20, 80, 12]] Original lists: [['a', 'b'], ['b', 'c', 'd'], ['e', 'f']] [['p', 'q'], ['p', 's', 't'], ['u', 'v', 'w']] Join the said two lists element wise: [['a', 'b', 'p', 'q'], ['b', 'c', 'd', 'p', 's', 't'], ['e', 'f', 'u', 'v', 'w']]
Flowchart:
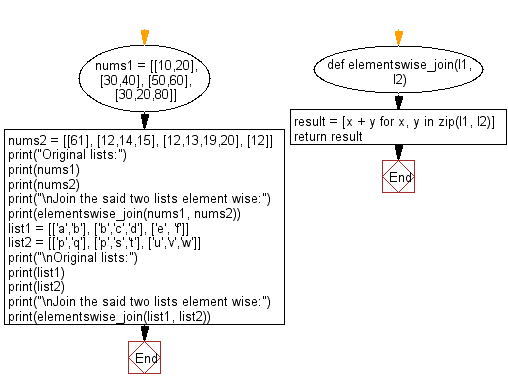
For more Practice: Solve these Related Problems:
- Write a Python program to join two lists of lists element-wise by aligning based on the shortest inner list length.
- Write a Python program to merge two lists of lists element-wise by padding shorter lists with a default value.
- Write a Python program to combine two lists of lists element-wise and then flatten the resulting structure into a single list.
- Write a Python program to interlace two lists of lists element-wise where one list contains numbers and the other contains strings, concatenating corresponding elements.
Python Code Editor:
Previous: Write a Python program to check if a given element occurs at least n times in a list.
Next: Write a Python program to add two given lists of different lengths, start from left.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics