Python: Generate and print a list of first and last 5 elements where the values are square of numbers between two numbers
Generate Square Numbers in Range
Write a Python program to generate and print a list of the first and last 5 elements where the values are square numbers between 1 and 30 (both included).
Visual Presentation:
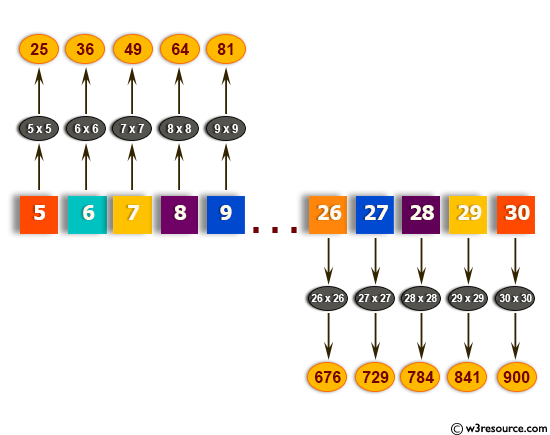
Sample Solution:
Python Code:
# Define a function named printValues
def printValues():
# Create an empty list 'l'
l = list()
# Loop from 1 to 20 (inclusive)
for i in range(1, 21):
# Calculate the square of 'i' and append it to the list 'l'
l.append(i**2)
# Print the first 5 elements of the list 'l'
print(l[:5])
# Print the last 5 elements of the list 'l'
print(l[-5:])
# Call the printValues function to execute it
printValues()
Sample Output:
[1, 4, 9, 16, 25] [256, 289, 324, 361, 400]
Explanation:
In the above example -
def printValues(): -> Defines a function named printValues.
l = list() -> Initializes an empty list named l.
for i in range(1,21): l.append(i**2)
Iterates through numbers 1 to 20 using a for loop and calculates their square, then appends the result to the l list.
print(l[:5]) -> Prints the first five elements of the l list using slice notation.
print(l[-5:]) -> Prints the last five elements of the l list using slice notation.
printValues() -> Finally calls the printValues() function. When executed, it will print the first five elements ([1, 4, 9, 16, 25]) and the last five elements ([256, 289, 324, 361, 400]) of the list of squares of numbers from 1 to 20.
Flowchart:
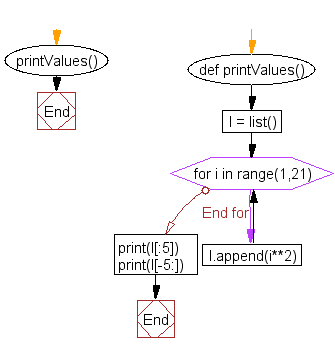
For more Practice: Solve these Related Problems:
- Write a Python program to generate a list of all cube numbers within a given range.
- Write a Python program to generate a list of numbers that are both perfect squares and perfect cubes.
- Write a Python program to generate a list of numbers whose square root is an odd number.
- Write a Python program to generate a list of numbers that are squares of prime numbers.
Go to:
Previous: Shuffle and print a specified list.
Next: Check if each number is prime in a list of numbers.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.