Python: Index of the first element which is greater than a specified element
Python List: Exercise - 163 with Solution
Write a Python program to get the index of the first element that is greater than a specified element.
Pictorial Presentation:
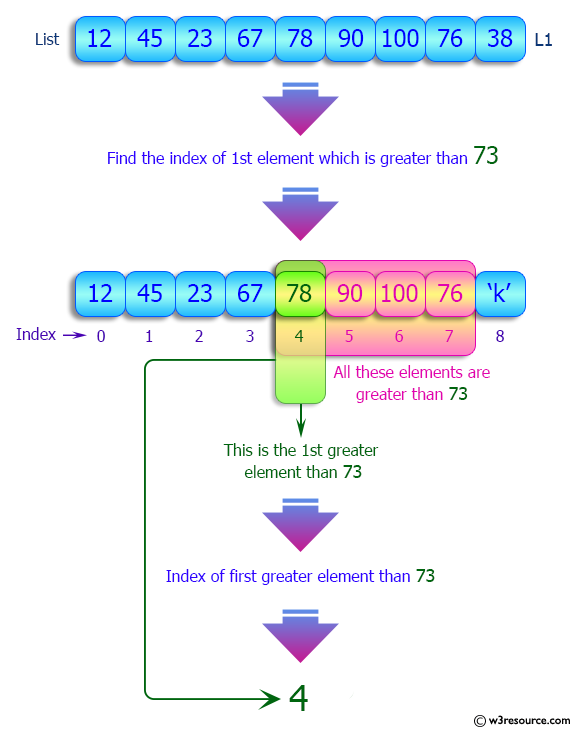
Sample Solution:
Python Code:
# Define a function called 'first_index' that finds the index of the first element in a list 'l1' greater than a given number 'n'.
def first_index(l1, n):
# Use the 'enumerate' function to iterate over the list 'l1' along with its indices.
# Find the first element in the list greater than 'n' and return its index.
return next(a[0] for a in enumerate(l1) if a[1] > n)
# Create a list 'nums' containing integer values.
nums = [12, 45, 23, 67, 78, 90, 100, 76, 38, 62, 73, 29, 83]
# Print a message indicating the original list.
print("Original list:")
print(nums)
# Set the value 'n' to 73 and print a message indicating the index of the first element greater than 'n' in the list.
n = 73
print("\nIndex of the first element which is greater than", n, "in the said list:")
print(first_index(nums, n))
# Set the value 'n' to 21 and print a message indicating the index of the first element greater than 'n' in the list.
n = 21
print("\nIndex of the first element which is greater than", n, "in the said list:")
print(first_index(nums, n))
# Set the value 'n' to 80 and print a message indicating the index of the first element greater than 'n' in the list.
n = 80
print("\nIndex of the first element which is greater than", n, "in the said list:")
print(first_index(nums, n))
# Set the value 'n' to 55 and print a message indicating the index of the first element greater than 'n' in the list.
n = 55
print("\nIndex of the first element which is greater than", n, "in the said list:")
print(first_index(nums, n))
Sample Output:
Original list: [12, 45, 23, 67, 78, 90, 100, 76, 38, 62, 73, 29, 83] Index of the first element which is greater than 73 in the said list: 4 Index of the first element which is greater than 21 in the said list: 1 Index of the first element which is greater than 80 in the said list: 5 Index of the first element which is greater than 55 in the said list: 3
Flowchart:
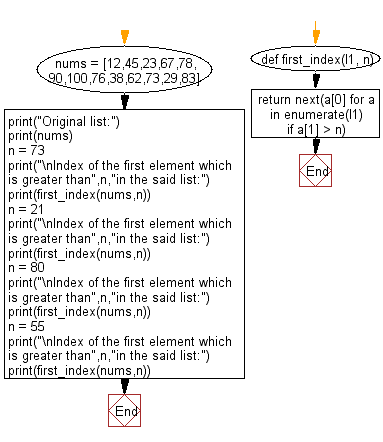
Python Code Editor:
Previous: Write a Python program to find the last occurrence of a specified item in a given list.
Next: Write a Python program to get the items from a given list with specific condition.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/list/python-data-type-list-exercise-163.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics