Python: Display each element vertically of a given list, list of lists
Python List: Exercise - 168 with Solution
Write a Python program to display vertically each element of a given list, list of lists.
Visual Presentation:
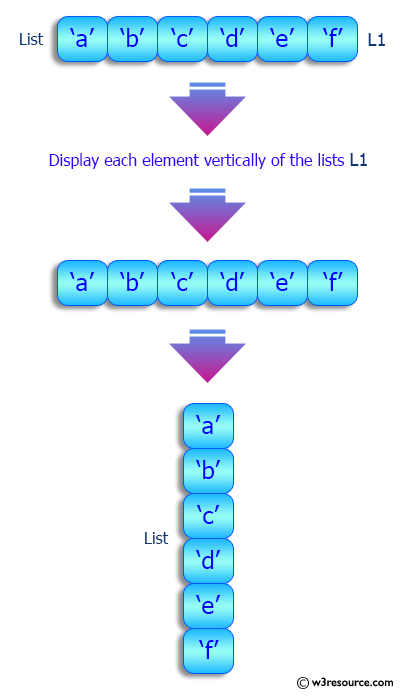
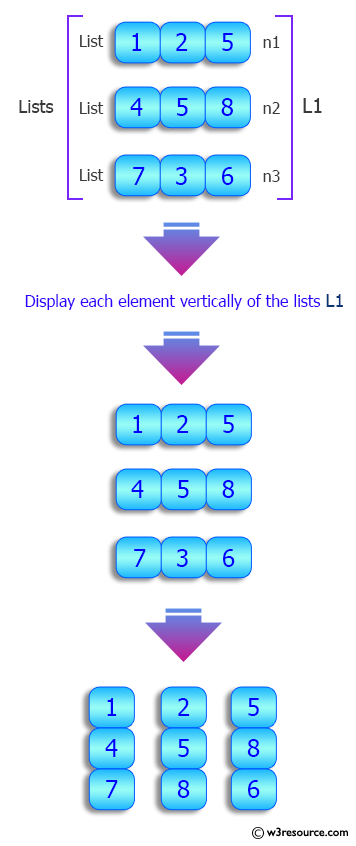
Sample Solution:
Python Code:
# Create a list 'text' containing string elements.
text = ["a", "b", "c", "d", "e", "f"]
# Print a message indicating the original list.
print("Original list:")
print(text)
# Print a message indicating the intention to display each element of the list vertically.
print("\nDisplay each element vertically of the said list:")
# Iterate through the elements in the list 'text' and print each element on a separate line.
for i in text:
print(i)
# Create a list of lists 'nums' containing sublists of integers.
nums = [[1, 2, 5], [4, 5, 8], [7, 3, 6]]
# Print a message indicating the original list of lists.
print("Original list:")
print(nums)
# Print a message indicating the intention to display each element of the list of lists vertically.
print("\nDisplay each element vertically of the said list of lists:")
# Use 'zip' and tuple unpacking to iterate through and print the elements in sublists vertically.
for a, b, c in zip(*nums):
print(a, b, c)
Sample Output:
Original list: ['a', 'b', 'c', 'd', 'e', 'f'] Display each element vertically of the said list: a b c d e f Original list: [[1, 2, 5], [4, 5, 8], [7, 3, 6]] Display each element vertically of the said list of lists: 1 4 7 2 5 3 5 8 6
Flowchart:
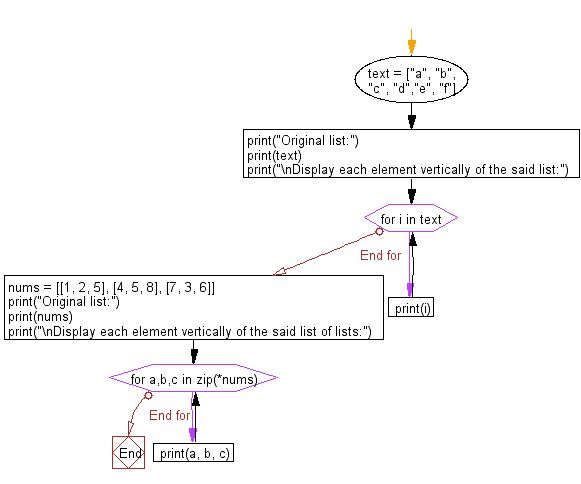
Python Code Editor:
Previous: Write a Python program to convert a given list of strings into list of lists.
Next: Write a Python program to convert a given list of strings and characters to a single list of characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/list/python-data-type-list-exercise-168.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics