Python: Convert a given list of strings and characters to a single list of characters
Convert Strings to List of Characters
Write a Python program to convert a given list of strings and characters to a single list of characters.
Visual Presentation:
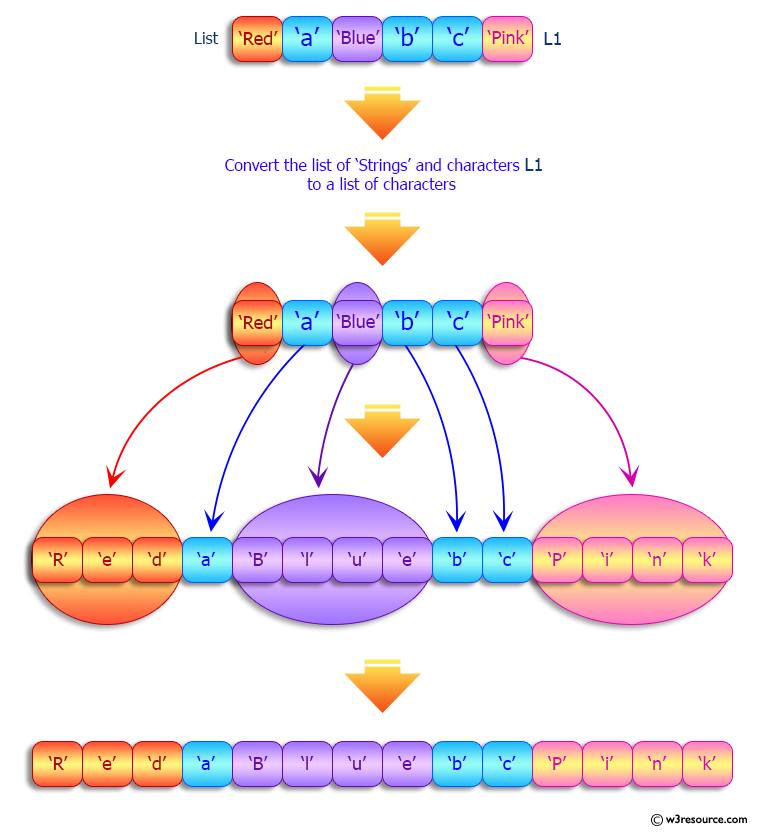
Sample Solution:
Python Code:
# Define a function called 'l_strs_to_l_chars' that converts a list of strings and characters into a single list of characters.
def l_strs_to_l_chars(lst):
# Use a nested list comprehension to create a new list 'result' where each character of the input elements is a separate element in the list.
result = [i for element in lst for i in element]
return result
# Create a list 'colors' containing string and character elements.
colors = ["red", "white", "a", "b", "black", "f"]
# Print a message indicating the original list.
print("Original list:")
print(colors)
# Print a message indicating the intention to convert the list of strings and characters into a single list of characters.
print("\nConvert the said list of strings and characters to a single list of characters:")
# Call the 'l_strs_to_l_chars' function to perform the conversion and print the result.
print(l_strs_to_l_chars(colors))
Sample Output:
Original list: ['red', 'white', 'a', 'b', 'black', 'f'] Convert the said list of strings and characters to a single list of characters: ['r', 'e', 'd', 'w', 'h', 'i', 't', 'e', 'a', 'b', 'b', 'l', 'a', 'c', 'k', 'f']
Flowchart:
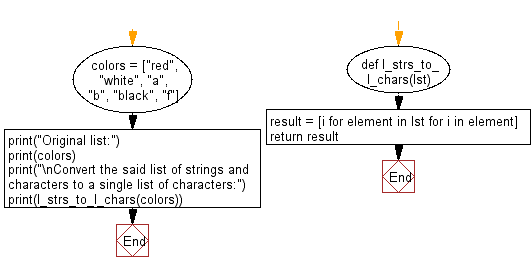
For more Practice: Solve these Related Problems:
- Write a Python program to flatten a mixed list of strings and individual characters into a single list of characters while preserving the order.
- Write a Python program to convert a list containing both strings and lists of characters into one consolidated list of characters without duplicates.
- Write a Python program to convert a nested list of strings into a flat list of characters, preserving the original sequence.
- Write a Python program to merge a list of strings and individual characters into a single list of characters, reversing each string before merging.
Go to:
Previous: Write a Python program to display vertically each element of a given list, list of lists.
Next: Write a Python program to insert an element in a given list after every nth position.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.