Python: Remove the last N number of elements from a given list
Remove Last N Elements from List
Write a Python program to remove the last N number of elements from a given list.
Visual Presentation:
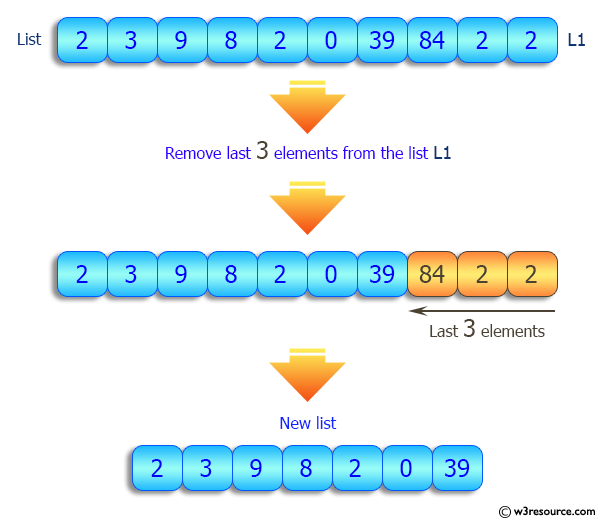
Flowchart:
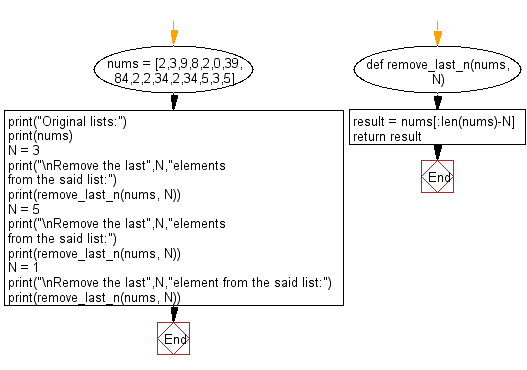
Sample Solution:
Python Code:
# Define a function called 'remove_last_n' that removes the last 'N' elements from a list 'nums'.
def remove_last_n(nums, N):
# Slice the list 'nums' to exclude the last 'N' elements and create a new list 'result'.
result = nums[:len(nums)-N]
return result
# Create a list 'nums' containing integer values.
nums = [2, 3, 9, 8, 2, 0, 39, 84, 2, 2, 34, 2, 34, 5, 3, 5]
# Print a message indicating the original list.
print("Original lists:")
print(nums)
# Set the value 'N' to 3 and print a message indicating the intention to remove the last 'N' elements from the list.
N = 3
print("\nRemove the last", N, "elements from the said list:")
# Call the 'remove_last_n' function to remove the last 3 elements from the list 'nums' and print the result.
print(remove_last_n(nums, N))
# Set the value 'N' to 5 and print a message indicating the intention to remove the last 'N' elements from the list.
N = 5
print("\nRemove the last", N, "elements from the said list:")
# Call the 'remove_last_n' function to remove the last 5 elements from the list 'nums' and print the result.
print(remove_last_n(nums, N))
# Set the value 'N' to 1 and print a message indicating the intention to remove the last 'N' element from the list.
N = 1
print("\nRemove the last", N, "element from the said list:")
# Call the 'remove_last_n' function to remove the last element from the list 'nums' and print the result.
print(remove_last_n(nums, N))
Sample Output:
Original lists: [2, 3, 9, 8, 2, 0, 39, 84, 2, 2, 34, 2, 34, 5, 3, 5] Remove the last 3 elements from the said list: [2, 3, 9, 8, 2, 0, 39, 84, 2, 2, 34, 2, 34] Remove the last 5 elements from the said list: [2, 3, 9, 8, 2, 0, 39, 84, 2, 2, 34] Remove the last 1 element from the said list: [2, 3, 9, 8, 2, 0, 39, 84, 2, 2, 34, 2, 34, 5, 3]
For more Practice: Solve these Related Problems:
- Write a Python program to remove the last N elements from a list and return the removed portion as a new list.
- Write a Python program to remove the last N elements only if they are even numbers.
- Write a Python program to remove the last N elements from a list when they form an ascending sequence.
- Write a Python program to remove the last N elements from a list and append a given value to the remainder.
Go to:
Previous: Write a Python program to concatenate element-wise three given lists.
Next: Write a Python program to merge some list items in given list using index value.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.