Python: Find the minimum, maximum value for each tuple position in a given list of tuples
Min and Max of Tuple Positions
Write a Python program to find the minimum and maximum value for each tuple position in a given list of tuples.
Sample Solution:
Python Code:
# Define a function called 'max_min_list_tuples' that computes the maximum and minimum values for each position in a list of tuples 'nums'.
def max_min_list_tuples(nums):
# Use 'zip' to transpose the tuples in 'nums' so that we can work with each position separately.
zip(*nums)
# Use 'map' and 'max' to find the maximum value for each position in the transposed tuples.
result1 = map(max, zip(*nums))
# Use 'map' and 'min' to find the minimum value for each position in the transposed tuples.
result2 = map(min, zip(*nums))
# Convert the results into lists and return them.
return list(result1), list(result2)
# Create a list of tuples 'nums' containing tuples of integer values.
nums = [(2, 3), (2, 4), (0, 6), (7, 1)]
# Print a message indicating the original list of tuples.
print("Original list:")
print(nums)
# Call the 'max_min_list_tuples' function to compute the maximum and minimum values for each tuple position in the list of tuples 'nums'.
result = max_min_list_tuples(nums)
# Print a message indicating the maximum value for each tuple position in the list of tuples.
print("\nMaximum value for each tuple position in the said list of tuples:")
print(result[0])
# Print a message indicating the minimum value for each tuple position in the list of tuples.
print("\nMinimum value for each tuple position in the said list of tuples:")
print(result[1])
Sample Output:
Original list: [(2, 3), (2, 4), (0, 6), (7, 1)] Maximum value for each tuple position in the said list of tuples: [7, 6] Minimum value for each tuple position in the said list of tuples: [0, 1]
Flowchart:
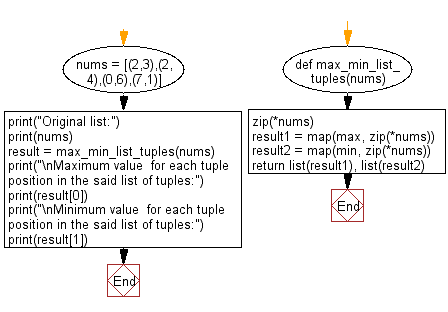
For more Practice: Solve these Related Problems:
- Write a Python program to calculate the sum of the minimum values for each position in a list of tuples.
- Write a Python program to determine the difference between the maximum and minimum values at each tuple position.
- Write a Python program to compute the average value for each tuple position in a list of tuples.
- Write a Python program to count the occurrences of the maximum value in each tuple position across a list of tuples.
Python Code Editor:
Previous: Write a Python program to add a number to each element in a given list of numbers.
Next: Write a Python program to create a new list dividing two given lists of numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics