Python: Iterate a given list cyclically on specific index position
Iterate List Cyclically at Index
Write a Python program to iterate a given list cyclically at a specific index position.
Visual Presentation:
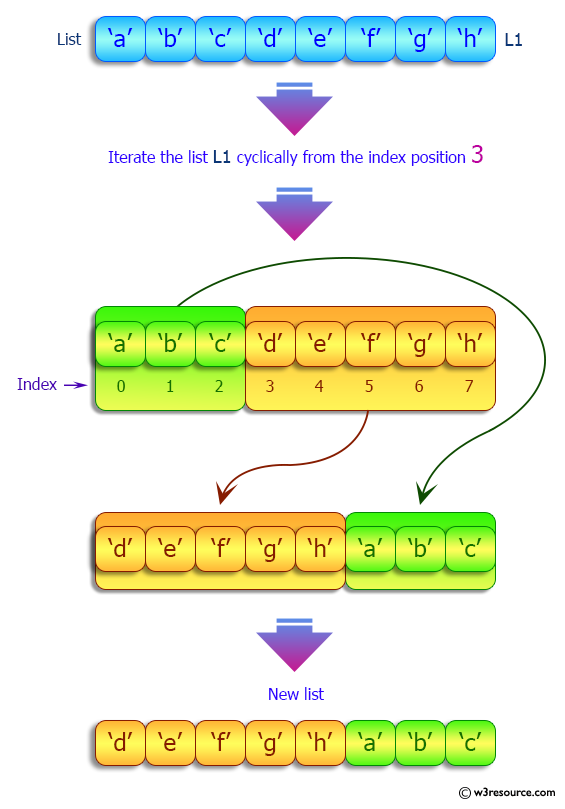
Sample Solution:
Python Code:
# Define a function called 'cyclically_iteration' that iterates a list cyclically based on a specific index position.
def cyclically_iteration(lst, spec_index):
result = [] # Initialize an empty list to store the cyclically iterated elements.
length = len(lst) # Get the length of the input list.
# Iterate through the list, starting from the 'spec_index' and wrapping around to the beginning if necessary.
for i in range(length):
element_index = spec_index % length # Calculate the index of the current element based on 'spec_index'.
result.append(lst[element_index]) # Append the element at the calculated index to the result list.
spec_index += 1 # Increment 'spec_index' for the next iteration, to move to the next element.
return result # Return the list of cyclically iterated elements.
# Create a list of characters 'chars'.
chars = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h']
# Print a message indicating the original list of characters.
print("Original list:")
print(chars)
# Specify a specific index position 'spec_index' for cyclic iteration and print a message.
spec_index = 3
print("\nIterate the said list cyclically on specific index position", spec_index, ":")
# Call the 'cyclically_iteration' function with 'chars' and 'spec_index' and print the result.
print(cyclically_iteration(chars, spec_index))
# Specify a different specific index position 'spec_index' for cyclic iteration and print a message.
spec_index = 5
print("\nIterate the said list cyclically on specific index position", spec_index, ":")
# Call the 'cyclically_iteration' function with 'chars' and the new 'spec_index' and print the result.
print(cyclically_iteration(chars, spec_index))
Sample Output:
Original list: ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h'] Iterate the said list cyclically on specific index position 3 : ['d', 'e', 'f', 'g', 'h', 'a', 'b', 'c'] Iterate the said list cyclically on specific index position 5 : ['f', 'g', 'h', 'a', 'b', 'c', 'd', 'e']
Flowchart:
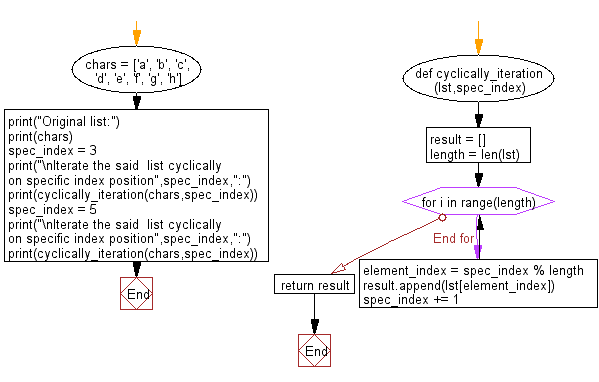
For more Practice: Solve these Related Problems:
- Write a Python program to cyclically iterate over a list starting from a specified index and return the reordered list.
- Write a Python program to cyclically rotate a list from a given index and then reverse only the rotated portion.
- Write a Python program to iterate a list cyclically at a specified index and interlace the result with the original order.
- Write a Python program to simulate a cyclic shift of list elements by k positions starting from a specific index.
Go to:
Previous: Write a Python program to create the smallest possible number using the elements of a given list of positive integers.
Next: Write a Python program to calculate the maximum and minimum sum of a sublist in a given list of lists.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.