Python: Maximum and minimum value of the three given lists
Python List: Exercise - 191 with Solution
Write a Python program to find the maximum and minimum values of the three given lists.
Visual Presentation:
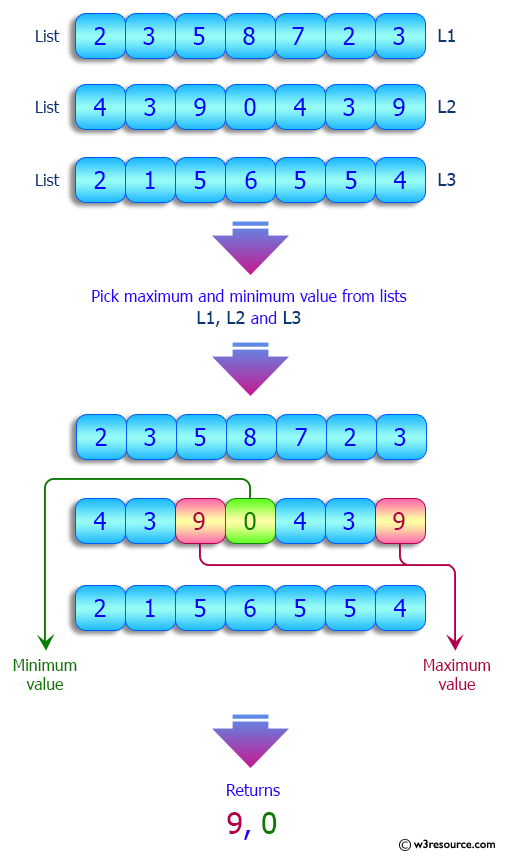
Sample Solution:
Python Code:
# Create three lists 'nums1', 'nums2', and 'nums3' containing integers.
nums1 = [2, 3, 5, 8, 7, 2, 3]
nums2 = [4, 3, 9, 0, 4, 3, 9]
nums3 = [2, 1, 5, 6, 5, 5, 4]
# Print a message indicating the original lists.
print("Original lists:")
# Print each of the three lists 'nums1', 'nums2', and 'nums3'.
print(nums1)
print(nums2)
print(nums3)
# Print a message indicating the maximum value of the three lists.
print("Maximum value of the said three lists:")
# Calculate the maximum value by combining all three lists and using the 'max' function.
print(max(nums1 + nums2 + nums3))
# Print a message indicating the minimum value of the three lists.
print("Minimum value of the said three lists:")
# Calculate the minimum value by combining all three lists and using the 'min' function.
print(min(nums1 + nums2 + nums3))
Sample Output:
Original lists: [2, 3, 5, 8, 7, 2, 3] [4, 3, 9, 0, 4, 3, 9] [2, 1, 5, 6, 5, 5, 4] Maximum value of the said three lists: 9 Minimum value of the said three lists: 0
Flowchart:
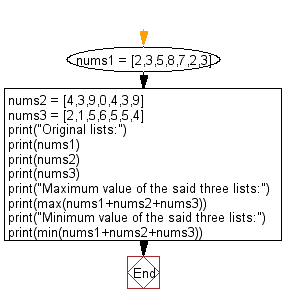
Python Code Editor:
Previous: Write a Python program to find the specified number of largest products from two given list, multiplying an element from each list.
Next: Write a Python program to remove all strings from a given list of tuples.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/list/python-data-type-list-exercise-191.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics