Python: Traverse a given list in reverse order, also print the elements with original index
Reverse List with Original Index
Write a Python program to traverse a given list in reverse order, and print the elements with the original index.
Visual Presentation:
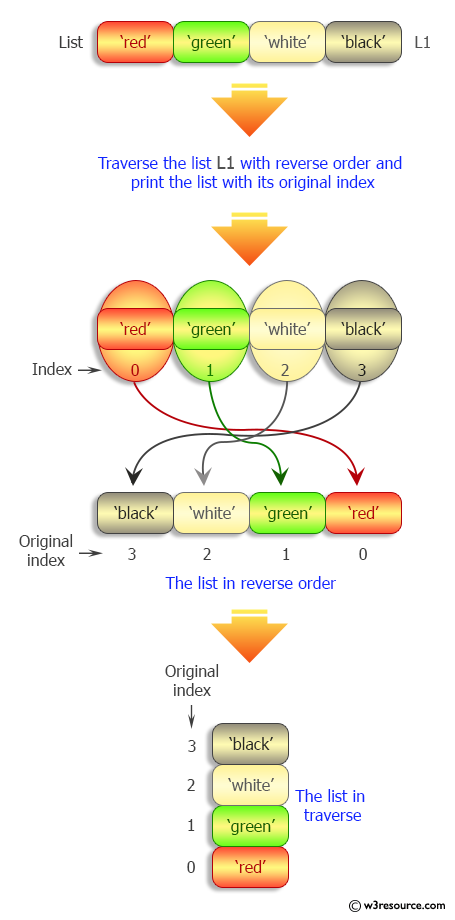
Sample Solution:
Python Code:
# Create a list called 'color' containing string elements.
color = ["red", "green", "white", "black"]
# Print a message indicating the original list of colors.
print("Original list:")
# Print the original 'color' list.
print(color)
# Print a message indicating the traversal of the list in reverse order.
print("\nTraverse the said list in reverse order:")
# Use the 'reversed' function to iterate over the 'color' list in reverse order.
# 'i' represents each color element in reverse order.
for i in reversed(color):
# Print each color element.
print(i)
# Print a message indicating the traversal of the list in reverse order with the original index.
print("\nTraverse the said list in reverse order with original index:")
# Use 'reversed' in conjunction with 'enumerate' to iterate over the 'color' list in reverse order.
# 'i' is the original index, and 'el' represents each color element in reverse order.
for i, el in reversed(list(enumerate(color))):
# Print the original index and the corresponding color element.
print(i, el)
Sample Output:
Original list: ['red', 'green', 'white', 'black'] Traverse the said list in reverse order: black white green red Traverse the said list in reverse order with original index: 3 black 2 white 1 green 0 red
Flowchart:
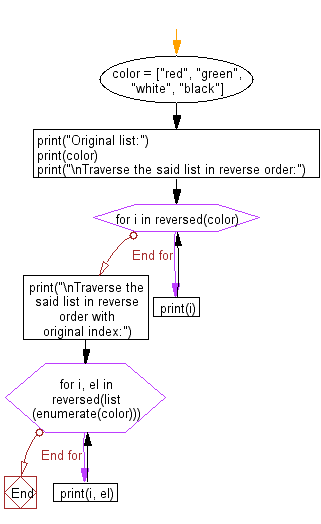
Python Code Editor:
Previous: Write a Python program to sum two or more lists, the lengths of the lists may be different.
Next: Write a Python program to move a specified element in a given list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics