Python: Move a specified element in a given list
Python List: Exercise - 196 with Solution
Write a Python program to move a specified element in a given list.
Visual Presentation:
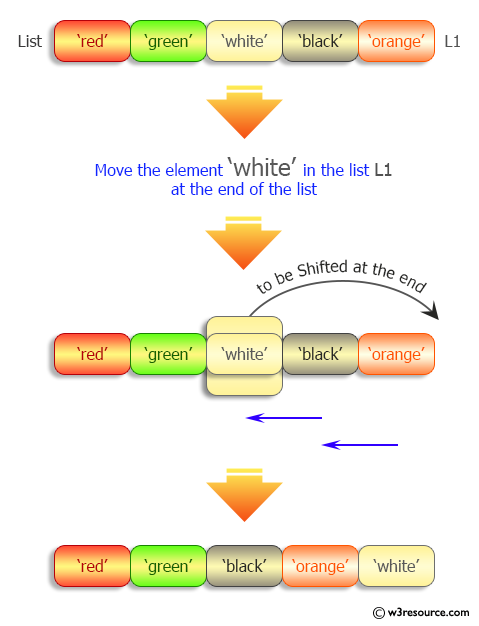
Sample Solution:
Python Code:
# Define a function 'group_similar_items' that moves a specified element to the end of a list.
def group_similar_items(seq, el):
# Remove the specified element 'el' from the list and append it to the end.
seq.append(seq.pop(seq.index(el)))
return seq # Return the modified list.
# Create a list 'colors' containing string elements.
colors = ['red', 'green', 'white', 'black', 'orange']
# Print a message indicating the original list of colors.
print("Original list:")
# Print the original 'colors' list.
print(colors)
# Specify the element 'el' to be moved to the end of the list.
el = "white"
# Print a message indicating the movement of 'el' to the end of the list.
print("Move", el, "at the end of the said list:")
# Call the 'group_similar_items' function with 'colors' and 'el', then print the result.
print(group_similar_items(colors, el))
# Revert to the original 'colors' list.
colors = ['red', 'green', 'white', 'black', 'orange']
# Print a message indicating the original list of colors.
print("\nOriginal list:")
# Print the original 'colors' list.
print(colors)
# Specify the element 'el' to be moved to the end of the list.
el = "red"
# Print a message indicating the movement of 'el' to the end of the list.
print("Move", el, "at the end of the said list:")
# Call the 'group_similar_items' function with 'colors' and 'el', then print the result.
print(group_similar_items(colors, el))
# Revert to the original 'colors' list.
colors = ['red', 'green', 'white', 'black', 'orange']
# Print a message indicating the original list of colors.
print("\nOriginal list:")
# Print the original 'colors' list.
print(colors)
# Specify the element 'el' to be moved to the end of the list.
el = "black"
# Print a message indicating the movement of 'el' to the end of the list.
print("Move", el, "at the end of the said list:")
# Call the 'group_similar_items' function with 'colors' and 'el', then print the result.
print(group_similar_items(colors, el))
Sample Output:
Original list: ['red', 'green', 'white', 'black', 'orange'] Move white at the end of the said list: ['red', 'green', 'black', 'orange', 'white'] Original list: ['red', 'green', 'white', 'black', 'orange'] Move red at the end of the said list: ['green', 'white', 'black', 'orange', 'red'] Original list: ['red', 'green', 'white', 'black', 'orange'] Move black at the end of the said list: ['red', 'green', 'white', 'orange', 'black']
Flowchart:
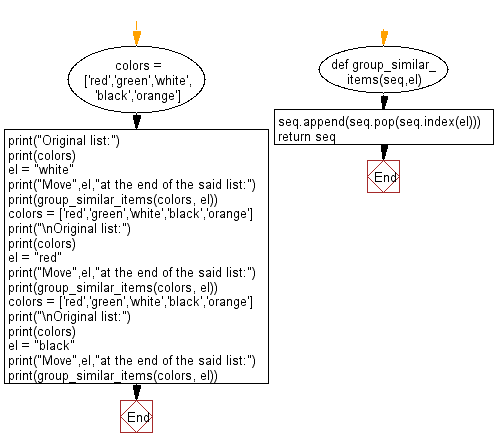
Python Code Editor:
Previous: Write a Python program to sum two or more lists, the lengths of the lists may be different.
Next: Write a Python program to compute the average of nth elements in a given list of lists with different lengths.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/list/python-data-type-list-exercise-196.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics