Python: Convert a given unicode list to a list contains strings
Convert Unicode List to Strings
Write a Python program to convert a Unicode list to a list of strings.
Sample Solution:
Python Code:
# Define a function 'unicode_to_str' that converts a list of unicode strings to a list of regular strings.
def unicode_to_str(lst):
# Use a list comprehension to iterate over each element 'x' in 'lst' and convert it to a regular string using 'str()'.
result = [str(x) for x in lst]
return result
# Create a list of unicode strings 'students'.
students = [u'S001', u'S002', u'S003', u'S004']
# Print a message indicating the original list.
print("Original lists:")
# Print the original list of unicode strings.
print(students)
# Print a message indicating the purpose of the following line of code.
print("Convert the said unicode list to a list containing strings:")
# Call the 'unicode_to_str' function to convert the list of unicode strings to a list of regular strings.
print(unicode_to_str(students))
Sample Output:
Original lists: ['S001', 'S002', 'S003', 'S004'] Convert the said unicode list to a list contains strings: ['S001', 'S002', 'S003', 'S004']
Flowchart:
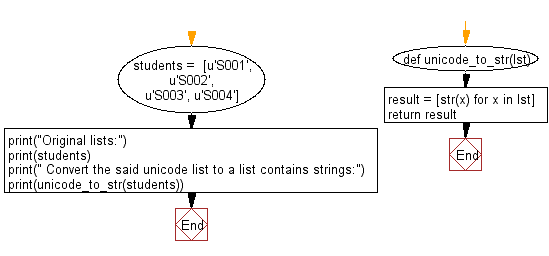
For more Practice: Solve these Related Problems:
- Write a Python program to convert a list of Unicode code points into their corresponding string characters.
- Write a Python program to convert a list of Unicode characters into one concatenated string.
- Write a Python program to convert a list of Unicode strings to a list of strings and filter out any that do not start with a letter.
- Write a Python program to convert a list of Unicode strings to ASCII strings, ignoring any non-ASCII characters.
Python Code Editor:
Previous: Write a Python program to compare two given lists and find the indices of the values present in both lists.
Next: Write a Python program to pair up the consecutive elements of a given list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.