Python: Multiply all the items in a list
Python List: Exercise-2 with Solution
Write a Python program to multiply all the items in a list.
Visual Presentation:
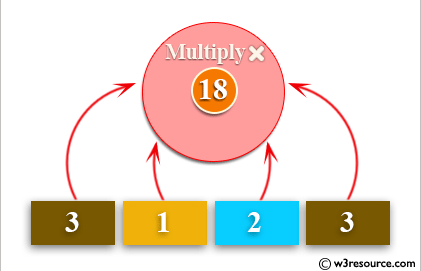
Sample Solution:
Python Code:
# Define a function called multiply_list that takes a list 'items' as input
def multiply_list(items):
# Initialize a variable 'tot' to store the product of the numbers, starting with 1
tot = 1
# Iterate through each element 'x' in the input list 'items'
for x in items:
# Multiply the current element 'x' with the 'tot' variable
tot *= x
# Return the final product of the numbers
return tot
# Call the multiply_list function with the list [1, 2, -8] as input and print the result
print(multiply_list([1, 2, -8]))
Sample Output:
-16
Explanation:
In the above exercise -
def multiply_list(items): -> This line defines a function called “multiply_list” that takes a single argument items. This function will be used to calculate the product of all the numbers in the items list.
- tot = 1 -> This line initializes a variable called tot to 1. This will be used to keep track of the running total of the product of the numbers in the list.
- for x in items: -> This line starts a loop that will iterate over each element in the items list, one at a time.
- tot *= x -> This line multiplies the current value of x by the tot variable. This is equivalent to the shorter form tot = tot * x.
- return tot -> This line returns the final value of the tot variable after the loop has finished. This is the product of all the numbers in the items list.
print(multiply_list([1,2,-8])) -> This line calls the multiply_list() function and passes in the list [1,2,-8]. The resulting product is then printed to the console using the print function.
Flowchart:
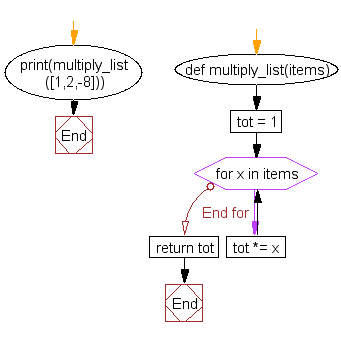
Python Code Editor:
Previous: Write a Python program to sum all the items in a list.
Next: Write a Python program to get the largest number from a list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/list/python-data-type-list-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics