Python: Check if a given string contains an element, which is present in a list
Check String Contains List Element
Write a Python program to check if a given string contains an element that is present in a list.
Visual Presentation:
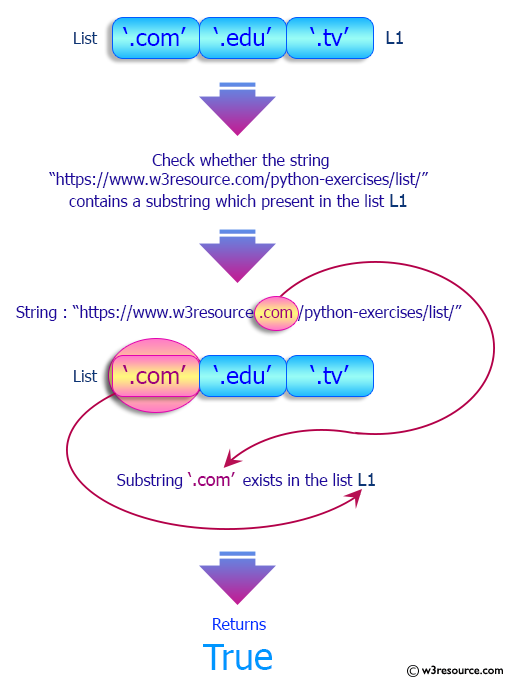
Sample Solution:
Python Code:
# Define a function 'test' that checks if any element in a list is present in a given string.
def test(lst, str1):
# Use a list comprehension to iterate over each element 'el' in the list 'lst'.
# For each 'el', check if it is present in the string 'str1'.
result = [el for el in lst if (el in str1)]
# Return a boolean indicating whether the 'result' list is not empty (i.e., if any element was found in the string).
return bool(result)
# Define a string 'str1' and a list 'lst' containing substrings.
str1 = "https://www.w3resource.com/python-exercises/list/"
lst = ['.com', '.edu', '.tv']
# Print a message indicating the original string and list.
print("The original string and list:")
# Print the original string.
print(str1)
# Print the list of substrings.
print(lst)
# Print a message indicating the purpose of the following lines of code.
print("\nCheck if", str1, "contains an element, which is present in the list", lst)
# Call the 'test' function to check if any element from the list 'lst' is present in the string 'str1'.
print(test(lst, str1))
# Define a string 'str1' and a list 'lst' containing substrings.
str1 = "https://www.w3resource.net"
lst = ['.com', '.edu', '.tv']
# Print a message indicating the original string and list.
print("\nThe original string and list: " + str1)
# Print the original string.
print(str1)
# Print the list of substrings.
print(lst)
# Print a message indicating the purpose of the following lines of code.
print("\nCheck if", str1, "contains an element, which is present in the list", lst)
# Call the 'test' function to check if any element from the list 'lst' is present in the string 'str1'.
print(test(lst, str1))
Sample Output:
The original string and list: https://www.w3resource.com/python-exercises/list/ ['.com', '.edu', '.tv'] Check if https://www.w3resource.com/python-exercises/list/ contains an element, which is present in the list ['.com', '.edu', '.tv'] True The original string and list: https://www.w3resource.net https://www.w3resource.net ['.com', '.edu', '.tv'] Check if https://www.w3resource.net contains an element, which is present in the list ['.com', '.edu', '.tv'] False
Flowchart:
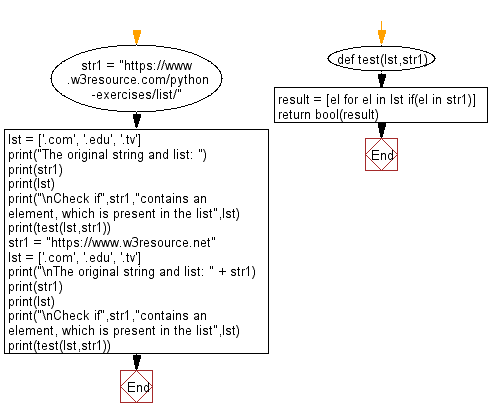
For more Practice: Solve these Related Problems:
- Write a Python program to check if a given string ends with any element from a provided list.
- Write a Python program to find and return the first element from a list that occurs as a substring in a given string.
- Write a Python program to verify if a string contains at least two distinct elements from a specified list.
- Write a Python program to check if all elements in a list appear as substrings within a given string.
Go to:
Previous: Write a Python program to pair up the consecutive elements of a given list.
Next: Write a Python program to find the indexes of all None items in a given list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.