Python: Join adjacent members of a given list
Python List: Exercise - 203 with Solution
Write a Python program to join adjacent members of a given list.
Visual Presentation:
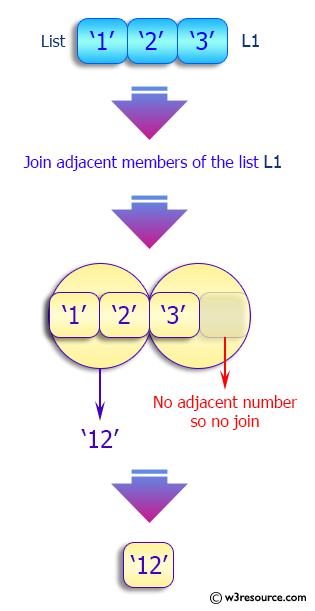
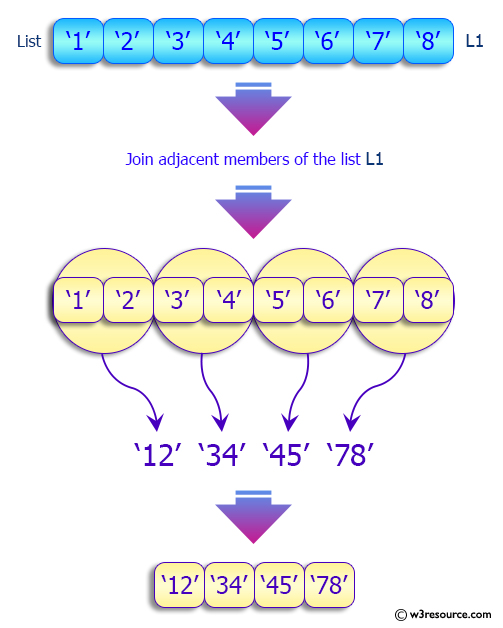
Sample Solution:
Python Code:
# Define a function 'test' that joins adjacent members of a given list.
def test(lst):
# Use list comprehensions to iterate over the list, taking elements at even and odd positions.
# Add the adjacent elements together and store the results in the 'result' list.
result = [x + y for x, y in zip(lst[::2], lst[1::2])]
# Return the 'result' list with joined adjacent members.
return result
# Define a list 'nums' containing string elements.
nums = ['1', '2', '3', '4', '5', '6', '7', '8']
# Print a message indicating the original list.
print("Original list:")
# Print the original list 'nums'.
print(nums)
# Print a message indicating the purpose of the following lines of code.
print("\nJoin adjacent members of a given list:")
# Call the 'test' function to join adjacent members of the list 'nums' and print the result.
print(test(nums))
# Define a new list 'nums' with fewer elements.
nums = ['1', '2', '3']
# Print a message indicating the original list.
print("\nOriginal list:")
# Print the new list 'nums'.
print(nums)
# Print a message indicating the purpose of the following lines of code.
print("\nJoin adjacent members of a given list:")
# Call the 'test' function again with the new list 'nums' and print the result.
print(test(nums))
Sample Output:
Original list: ['1', '2', '3', '4', '5', '6', '7', '8'] Join adjacent members of a given list: ['12', '34', '56', '78'] Original list: ['1', '2', '3'] Join adjacent members of a given list: ['12']
Flowchart:
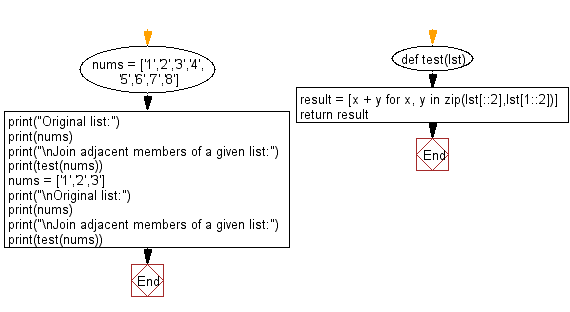
Python Code Editor:
Previous: Write a Python program to find the indexes of all None items in a given list.
Next: Write a Python program to check if first digit/character of each element in a given list is same or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/list/python-data-type-list-exercise-203.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics