Python: Compute the sum of non-zero groups (separated by zeros) of a given list of numbers
Sum of Non-Zero Groups
Write a Python program to compute the sum of non-zero groups (separated by zeros) of a given list of numbers.
Sample Solution:
Python Code:
# Define a function 'test' that computes the sum of non-zero groups separated by zeros in a list.
def test(lst):
# Initialize an empty list 'result' to store the sums of non-zero groups.
result = []
# Initialize a variable 'ele_val' to keep track of the current group's sum.
ele_val = 0
# Iterate through the elements in the input list 'lst'.
for digit in lst:
# Check if the current digit is zero.
if digit == 0:
# If 'ele_val' is not zero (meaning the end of a non-zero group),
# append it to the 'result' list and reset 'ele_val' to zero.
if ele_val != 0:
result.append(ele_val)
ele_val = 0
else:
# Add the current digit to the 'ele_val' to accumulate the group's sum.
ele_val += digit
# If 'ele_val' is greater than zero (meaning there's a non-zero group at the end of the list),
# append it to the 'result' list.
if ele_val > 0:
result.append(ele_val)
return result
# Define a list of numbers 'nums'.
nums = [3, 4, 6, 2, 0, 0, 0, 0, 0, 0, 6, 7, 6, 9, 10, 0, 0, 0, 0, 0, 7, 4, 4, 0, 0, 0, 0, 0, 0, 5, 3, 2, 9, 7, 1, 0, 0, 0]
# Print a message indicating the original list.
print("\nOriginal list:")
# Print the original list of numbers 'nums'.
print(nums)
# Print a message indicating the purpose of the following lines of code.
print("\nCompute the sum of non-zero groups (separated by zeros) of the said list of numbers:")
# Call the 'test' function to compute the sums of non-zero groups in 'nums' and print the result.
print(test(nums))
Sample Output:
Original list: [3, 4, 6, 2, 0, 0, 0, 0, 0, 0, 6, 7, 6, 9, 10, 0, 0, 0, 0, 0, 7, 4, 4, 0, 0, 0, 0, 0, 0, 5, 3, 2, 9, 7, 1, 0, 0, 0] Compute the sum of non-zero groups (separated by zeros) of the said list of numbers: [15, 38, 15, 27]
Flowchart:
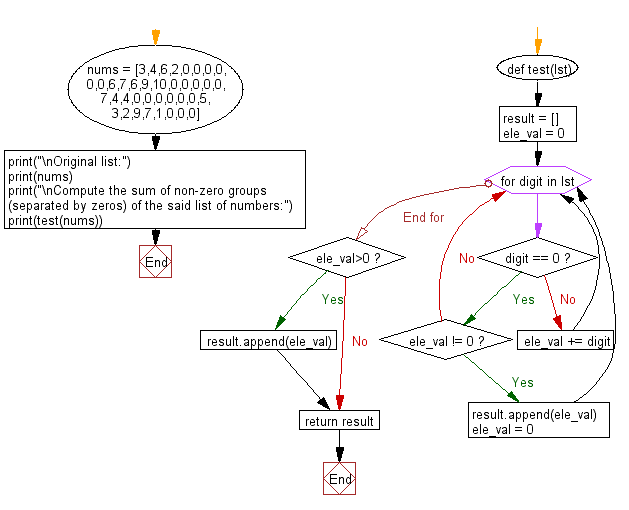
For more Practice: Solve these Related Problems:
- Write a Python program to compute the product of non-zero groups (separated by zeros) in a list.
- Write a Python program to calculate both the sum and average of each non-zero group within a list.
- Write a Python program to find the non-zero group with the maximum sum and return its elements.
- Write a Python program to compute the sum of non-zero groups and then sort these groups by their sums.
Python Code Editor:
Previous: Write a Python program to count the number of groups of non-zero numbers separated by zeros of a given list of numbers.
Next: Write a Python program to remove an element from a given list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics