Python: Remove all the values except integer values from a given array of mixed values
Filter Integers from Mixed List
Write a Python program to remove all values except integer values from a given array of mixed values.
Sample Solution-1:
Python Code:
# Define a function called 'test' that filters a list to include only integer values.
def test(lst):
return [lst for lst in lst if isinstance(lst, int)]
# Create a list 'mixed_list' containing a mix of data types, including floats, integers, and strings.
mixed_list = [34.67, 12, -94.89, "Python", 0, "C#"]
# Print a message indicating the original list.
print("Original list:", mixed_list)
# Print a message indicating the purpose of the following lines of code.
print("After removing all the values except integer values from the said array of mixed values:")
# Call the 'test' function with 'mixed_list' and print the result, which contains only integer values.
print(test(mixed_list))
Sample Output:
Original list: [34.67, 12, -94.89, 'Python', 0, 'C#'] After removing all the values except integer values from the said array of mixed values: [12, 0]
Flowchart:
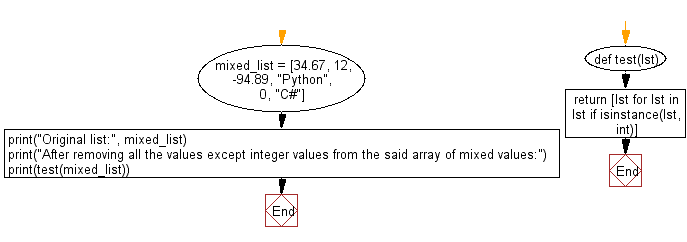
Sample Solution-2:
Python Code:
# Define a function called 'test' that filters a list to include only values of integer type.
def test(lst):
return [x for x in lst if type(x) == int]
# Create a list 'mixed_list' containing a mix of data types, including floats, integers, and strings.
mixed_list = [34.67, 12, -94.89, "Python", 0, "C#"]
# Print a message indicating the original list.
print("Original list:", mixed_list)
# Print a message indicating the purpose of the following lines of code.
print("After removing all the values except integer values from the said array of mixed values:")
# Call the 'test' function with 'mixed_list' and print the result, which contains only integer values.
print(test(mixed_list))
Sample Output:
Original list: [34.67, 12, -94.89, 'Python', 0, 'C#'] After removing all the values except integer values from the said array of mixed values: [12, 0]
Flowchart:
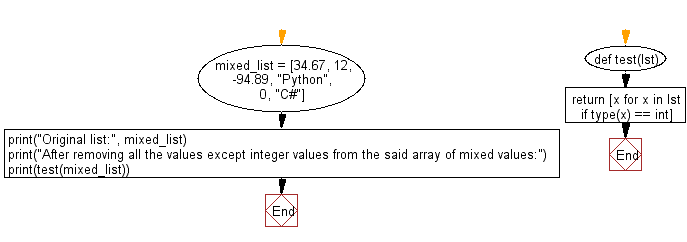
For more Practice: Solve these Related Problems:
- Write a Python program to extract only the string elements from a mixed list.
- Write a Python program to filter out all non-integer values from a list and convert numeric strings to integers.
- Write a Python program to filter only the floating-point numbers from a mixed list.
- Write a Python program to extract all integer values from a nested list containing mixed types.
Go to:
Previous: Write a Python program to remove an element from a given list.
Next: Write a Python program to calculate the sum of two lowest negative numbers of a given array of integers.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.