Python: Sort a given positive number in descending/ascending order
Sort Number Ascending/Descending
Write a Python program to sort a given positive number in descending/ascending order.
Descending -> Highest to lowest.
Ascending -> Lowest to highest
Sample Solution:
Python Code:
# Define a function called 'test_dsc' that takes an integer 'n' and returns the integer formed by its digits sorted in descending order.
def test_dsc(n):
# Convert the integer 'n' to a string, sort its characters in descending order, and convert them back to an integer.
return int(''.join(sorted(str(n), reverse=True)))
# Define a function called 'test_asc' that takes an integer 'n' and returns the integer formed by its digits sorted in ascending order.
def test_asc(n):
# Convert the integer 'n' to a string, sort its characters in ascending order, and convert them back to an integer.
return int(''.join(sorted(list(str(n)))[::1]))
# Assign an integer value to 'n'.
n = 134543
# Print a message indicating the original number.
print("Original Number: ", n)
# Calculate and print the descending order of the number using the 'test_dsc' function.
print("Descending order of the said number: ", test_dsc(n))
# Calculate and print the ascending order of the number using the 'test_asc' function.
print("Ascending order of the said number: ", test_asc(n))
# Assign another integer value to 'n'.
n = 43750973
# Print a message indicating the original number.
print("\nOriginal Number: ", n)
# Calculate and print the descending order of the number using the 'test_dsc' function.
print("Descending order of the said number: ", test_dsc(n))
# Calculate and print the ascending order of the number using the 'test_asc' function.
print("Ascending order of the said number: ", test_asc(n))
Sample Output:
Original Number: 134543 Descending order of the said number: 544331 Ascending order of the said number: 133445 Original Number: 43750973 Descending order of the said number: 97754330 Ascending order of the said number: 3345779
Flowchart:
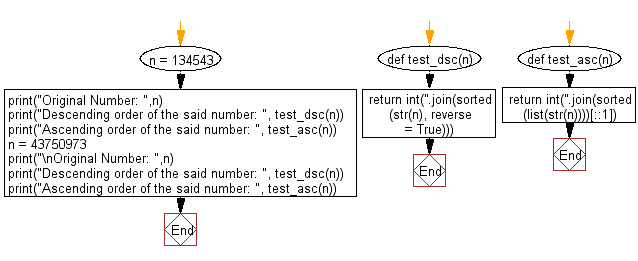
For more Practice: Solve these Related Problems:
- Write a Python program to sort the digits of a number in alternating ascending and descending order.
- Write a Python program to sort a positive number’s digits in ascending order and then convert the result back to an integer.
- Write a Python program to sort a number’s digits such that all even digits appear first in descending order followed by odd digits in ascending order.
- Write a Python program to sort the digits of a number in descending order and then calculate the difference between the original number and the sorted number.
Go to:
Previous: Write a Python program to calculate the sum of two lowest negative numbers of a given array of integers.
Next: Write a Python program to merge two or more lists into a list of lists, combining elements from each of the input lists based on their positions.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.