Python: Map the values of a list to a dictionary using a function
Python List: Exercise - 220 with Solution
Write a Python program to map the values of a list to a dictionary using a function, where the key-value pairs consist of the original value as the key and the result of the function as the value.
- Use map() to apply fn to each value of the list.
- Use zip() to pair original values to the values produced by fn.
- Use dict() to return an appropriate dictionary.
Sample Solution:
Python Code:
# Define a function called 'map_dictionary' that takes an iterable 'itr' and a function 'fn' as arguments.
def map_dictionary(itr, fn):
# Create a dictionary by zipping the iterable 'itr' and the result of applying the function 'fn' to each element in 'itr'.
return dict(zip(itr, map(fn, itr)))
# Example usage: Use the 'map_dictionary' function to create a dictionary where the keys are elements from the list [1, 2, 3] and the values are the squares of those elements.
print(map_dictionary([1, 2, 3], lambda x: x * x))
Sample Output:
{1: 1, 2: 4, 3: 9}
Flowchart:
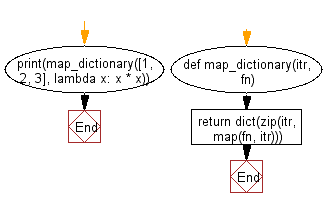
Python Code Editor:
Previous: Write a Python program to build a list, using an iterator function and an initial seed value.
Next: Write a Python program to randomize the order of the values of an list, returning a new list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/list/python-data-type-list-exercise-220.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics