Python: Retrieve the value of the nested key indicated by the given selector list from a dictionary or list
Retrieve Nested Key Value
Write a Python program to retrieve the value of the nested key indicated by the given selector list from a dictionary or list.
- Use functools.reduce() to iterate over the selectors list.
- Apply operator.getitem() for each key in selectors, retrieving the value to be used as the iteratee for the next iteration.
Sample Solution:
Python Code:
# Import the 'reduce' function from the 'functools' module and the 'getitem' function from the 'operator' module.
from functools import reduce
from operator import getitem
# Define a function called 'get' that takes a dictionary 'd' and a list of 'selectors'.
def get(d, selectors):
# Use the 'reduce' function to access nested dictionary values by applying 'getitem' successively.
return reduce(getitem, selectors, d)
# Create a nested dictionary 'users' with user information.
users = {
'freddy': {
'name': {
'first': 'Fateh',
'last': 'Harwood'
},
'postIds': [1, 2, 3]
}
}
# Example: Access values in the 'users' dictionary using nested selectors.
print(get(users, ['freddy', 'name', 'last']))
print(get(users, ['freddy', 'postIds', 1]))
Sample Output:
Harwood 2
Flowchart:
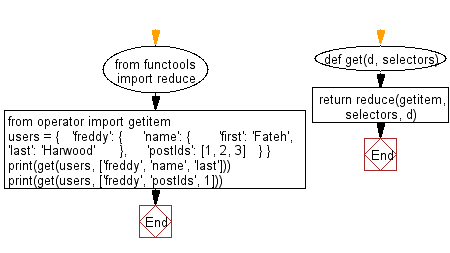
For more Practice: Solve these Related Problems:
- Write a Python program to retrieve a nested key value from a deeply nested dictionary using a list of keys, handling missing keys gracefully.
- Write a Python program to extract a nested value from a dictionary where the key path is provided as a dot-separated string.
- Write a Python program to retrieve nested key values from a list of dictionaries given a common selector list.
- Write a Python program to extract nested values from a mixed dictionary and list structure using recursion.
Go to:
Previous: Write a Python program to create a list with the unique values filtered out.
Next: Write a Python program to get a list of elements that exist in both lists, after applying the provided function to each list element of both.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.