Python: Get a list of elements that exist in both lists
Python List: Exercise - 226 with Solution
Write a Python program to get a list of elements that exist in both lists, after applying the provided function to each list element of both.
- Create a set, using map() to apply fn to each element in b.
- Use a list comprehension in combination with fn on a to only keep values contained in both lists.
Sample Solution-1:
Applying a given function:
Python Code:
# Import the necessary modules and functions.
from math import floor
# Define a function 'intersection_by' that takes two lists 'a' and 'b' and a function 'fn' as input.
def intersection_by(a, b, fn):
# Create a set '_b' containing the results of applying 'fn' to each element in list 'b'.
_b = set(map(fn, b))
# Return a list containing elements from list 'a' where 'fn(item)' is in the set '_b'.
return [item for item in a if fn(item) in _b]
# Provide an example of using 'intersection_by' function to find the intersection of two lists using 'floor' function as the key.
print(intersection_by([2.1, 1.2], [2.3, 3.4], floor))
Sample Output:
[2.1]
Flowchart:
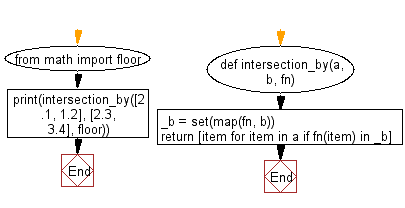
Sample Solution-2:
Without applying any function:
Use a list comprehension on x to only keep values contained in both lists.
Python Code:
# Define a function 'similarity' that takes two lists 'x' and 'y' as input.
def similarity(x, y):
# Return a list containing elements from list 'x' that also exist in list 'y'.
return [item for item in x if item in y]
# Define two lists 'nums1' and 'nums2'.
nums1 = [1, 2, 3, 4]
nums2 = [1, 2, 4, 5]
# Display a message and the original lists.
print("Original lists:")
print(nums1)
print(nums2)
# Display a message and the list of elements that exist in both lists using the 'similarity' function.
print("\nList of elements that exist in both lists:")
print(similarity(nums1, nums2))
Sample Output:
Original lists: [1, 2, 3, 4] [1, 2, 4, 5] List of elements that exist in both lists: [1, 2, 4]
Flowchart:
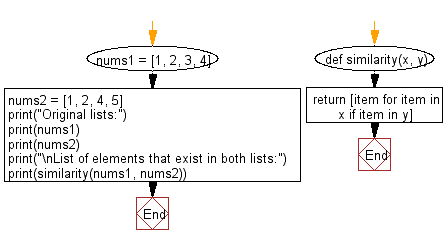
Sample Solution-3:
Without applying any function:
- Create a set from a and b.
- Use the built-in set operator & to only keep values contained in both sets, then transform the set back into a list.
Python Code:
# Define a function 'common_elements' that takes two lists 'l1' and 'l2' as input.
def common_elements(l1, l2):
# Convert 'l1' and 'l2' into sets to eliminate duplicate values.
_l1, _l2 = set(l1), set(l2)
# Find the intersection (common elements) between the sets and convert it back to a list.
return list(_l1 & _l2)
# Define two lists 'nums1' and 'nums2'.
nums1 = [1, 2, 3, 4]
nums2 = [1, 2, 4, 5]
# Display a message and the original lists.
print("Original lists:")
print(nums1)
print(nums2)
# Display a message and the list of elements that exist in both lists using the 'common_elements' function.
print("\nList of elements that exist in both lists:")
print(common_elements(nums1, nums2))
Sample Output:
Original lists: [1, 2, 3, 4] [1, 2, 4, 5] List of elements that exist in both lists: [1, 2, 4]
Flowchart:
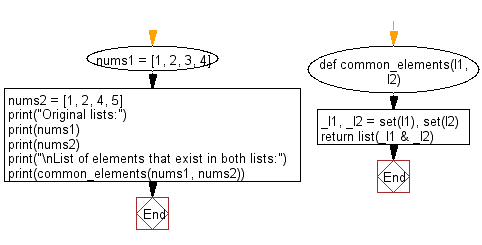
Python Code Editor:
Previous: Write a Python program to retrieve the value of the nested key indicated by the given selector list from a dictionary or list.
Next: Write a Python program to get the symmetric difference between two lists, after applying the provided function to each list element of both.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/list/python-data-type-list-exercise-226.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics