Python: Find the items that are parity outliers in a given list
Find Parity Outliers
Write a Python program to find items that are parity outliers in a given list.
- Use collections.Counter with a list comprehension to count even and odd values in the list.
- Use collections.Counter.most_common() to get the most common parity.
- Use a list comprehension to find all elements that do not match the most common parity.
Sample Solution:
Python Code:
# Import the 'Counter' class from the 'collections' module.
from collections import Counter
# Define a function 'find_parity_outliers' that takes a list of numbers 'nums' as input.
def find_parity_outliers(nums):
# Use a list comprehension to iterate through the numbers 'x' in 'nums'.
# For each number 'x', check if it has a different parity (odd or even) compared to the most common parity in the 'nums'.
# The 'most_common' method of 'Counter' returns a list of tuples where the first element is the most common parity (0 for even, 1 for odd) and the second element is the count.
# Return a list of numbers where the parity is different from the most common parity in 'nums'.
return [
x for x in nums
if x % 2 != Counter([n % 2 for n in nums]).most_common()[0][0]
]
# Call the 'find_parity_outliers' function with example lists of numbers and print the results.
print(find_parity_outliers([1, 2, 3, 4, 6]))
print(find_parity_outliers([1, 2, 3, 4, 5, 6, 7]))
Sample Output:
[1, 3] [2, 4, 6]
Flowchart:
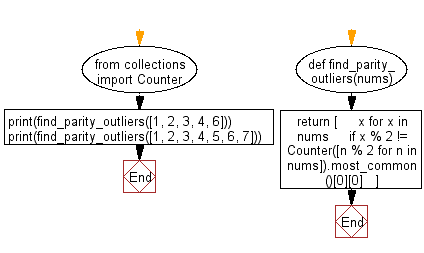
For more Practice: Solve these Related Problems:
- Write a Python program to identify the single parity outlier in a list where all but one number share the same even/odd status.
- Write a Python program to detect parity outliers in a list when multiple numbers deviate from the common parity.
- Write a Python program to determine if a list has a unique parity outlier and return both its value and index.
- Write a Python program to separate a list into even and odd numbers and then identify which group contains fewer elements.
Previous: Write a Python program to find the index of the last element in the given list that satisfies the provided testing function.
Next: Write a Python program to convert a given list of dictionaries into a list of values corresponding to the specified key.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.