Python: Find the value of the first element in the given list that satisfies the provided testing function
First Value Satisfying Function
Write a Python program to find the value of the first element in the given list that satisfies the provided testing function.
Use a list comprehension and next() to return the first element in lst for which fn returns True.
Sample Solution:
Python Code:
# Define a function 'find' that takes a list 'lst' and a function 'fn' as input.
def find(lst, fn):
# Use a generator expression to find the first element 'x' in the list 'lst' for which 'fn(x)' is true.
# The 'next' function is used to retrieve the first matching element.
return next(x for x in lst if fn(x))
# Call the 'find' function with a list and a lambda function to find the first odd number in the list.
# Print the result.
print(find([1, 2, 3, 4], lambda n: n % 2 == 1))
# Call the 'find' function again with a lambda function to find the first even number in the list.
# Print the result.
print(find([1, 2, 3, 4], lambda n: n % 2 == 0))
Sample Output:
1 2
Flowchart:
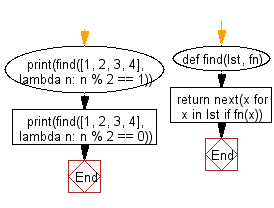
For more Practice: Solve these Related Problems:
- Write a Python program to find the first value in a list that satisfies a custom condition and return it, or None if no value qualifies.
- Write a Python program to retrieve the first value in a list that passes a provided test function and print its index.
- Write a Python program to find the first palindromic string in a list using a given testing function.
- Write a Python program to return the first value in a list that exceeds a specified threshold after applying a lambda function.
Go to:
Previous: Write a Python program to calculate the average of a given list, after mapping each element to a value using the provided function.
Next: Write a Python program to find the value of the last element in the given list that satisfies the provided testing function.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.