Python: Return the symmetric difference between two iterables, without filtering out duplicate values
Symmetric Difference with Duplicates
Write a Python program to get the symmetric difference between two iterables, without filtering out duplicate values.
- Create a set from each list.
- Use a list comprehension on each of them to only keep values not contained in the previously created set of the other.
Sample Solution:
Python Code:
# Define a function 'symmetric_difference' that takes two lists, 'x' and 'y', as input.
def symmetric_difference(x, y):
# Create sets '_x' and '_y' from the input lists 'x' and 'y'.
_x, _y = set(x), set(y)
# Calculate the symmetric difference of the two sets and store it in a list.
# The symmetric difference contains elements that are unique to each set.
symmetric_diff = [item for item in x if item not in _y] + [item for item in y if item not in _x]
# Return the result.
return symmetric_diff
# Call the 'symmetric_difference' function with two lists and print the symmetric difference.
print(symmetric_difference([10, 20, 30], [10, 20, 40]))
Sample Output:
[30, 40]
Flowchart:
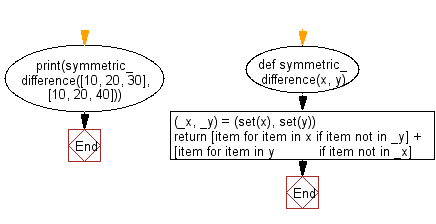
For more Practice: Solve these Related Problems:
- Write a Python program to compute the symmetric difference of two lists with duplicates while preserving the original order.
- Write a Python program to find the symmetric difference between two iterables including duplicate occurrences and output the result as a sorted list.
- Write a Python program to compute the symmetric difference with duplicates and also return a count of each differing element.
- Write a Python program to determine the symmetric difference between two lists after applying a transformation function to each element.
Go to:
Previous: Write a Python program to create a dictionary with the unique values of a given list as keys and their frequencies as the values.
Next: Write a Python program to check if a given function returns True for every element in a list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.