Python: Calculate the difference between two iterables, without filtering duplicate values
Difference Without Filtering Duplicates
Write a Python program to calculate the difference between two iterables, without filtering duplicate values.
- Create a set from y.
- Use a list comprehension on x to only keep values not contained in the previously created set, _y.
Sample Solution:
Python Code:
# Define a function 'difference' that takes two lists 'x' and 'y'.
# It converts 'y' into a set for faster membership checking.
# It returns a new list containing elements that are in 'x' but not in 'y'.
def difference(x, y):
_y = set(y)
return [item for item in x if item not in _y]
# Call the 'difference' function with two lists and print the result.
print(difference([1, 2, 3], [1, 2, 4]))
Sample Output:
[3]
Flowchart:
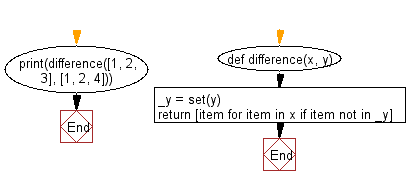
For more Practice: Solve these Related Problems:
- Write a Python program to compute the difference between two lists, preserving duplicate occurrences in the result.
- Write a Python program to calculate the difference between two iterables including duplicates, then output both the difference and the count of duplicates.
- Write a Python program to determine the difference between two lists without filtering duplicates and maintain the original order of appearance.
- Write a Python program to find elements present in one list but not in another, considering duplicate values.
Python Code Editor:
Previous: Write a Python program to check if a given function returns True for at least one element in the list.
Next: Write a Python program to get the maximum value of a list, after mapping each element to a value using a given function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics