Python: Select an item randomly from a list
Select Random Item from List
Write a Python program to select an item randomly from a list.
Use random.choice() to get a random element from a given list.
Example - 1 :
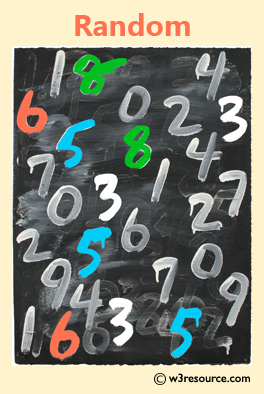
Example - 2 :
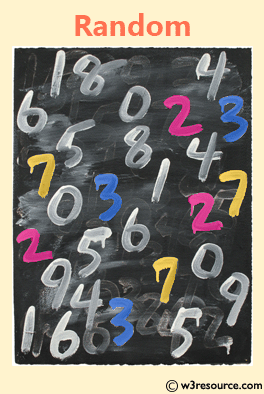
Example - 3 :
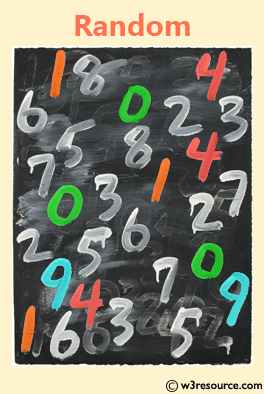
Sample Solution-1:
Python Code:
# Import the 'random' module, which provides functions for generating random values
import random
# Define a list 'color_list' containing various colors
color_list = ['Red', 'Blue', 'Green', 'White', 'Black']
# Use the 'random.choice' function to select and print a random color from the 'color_list'
print(random.choice(color_list))
Sample Output:
Black
Sample Solution-2:
Python Code:
# Import the 'choice' function from the 'random' module to select a random element from a list
from random import choice
# Define a function named 'random_element' that takes a list 'lst' as a parameter
def random_element(lst):
# Use the 'choice' function to return a random element from the input list 'lst'
return choice(lst)
# Call the 'random_element' function with a list as an argument and print the randomly selected element
print(random_element([2, 3, 4, 7, 9, 11, 15]))
Sample Output:
4
Flowchart:
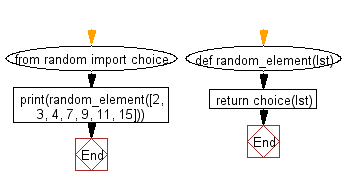
For more Practice: Solve these Related Problems:
- Write a Python program to randomly shuffle a list and return a new list.
- Write a Python program to randomly select multiple unique items from a list.
- Write a Python program to select an item randomly from a nested list.
- Write a Python program to select a random sublist of size n from a given list.
Go to:
Previous: Write a Python program to append a list to the second list.
Next: Write a python program to check whether two lists are circularly identical.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.