Python: Find the weighted average of two or more numbers
Weighted Average of Numbers
Write a Python program to get the weighted average of two or more numbers.
A weighted average is an average in which some of the items to be averaged are 'more important' or 'less important' than some of the others. The weights are (non-negative) numbers which measure the relative importance
For example, the weighted average of a list of numbers x1,…,xn with corresponding weights w1,…,wn is
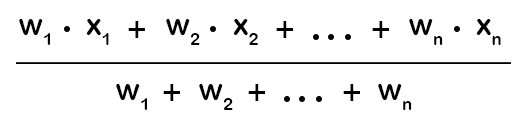
Ref: mathinsight.org
- Use sum() to sum the products of the numbers by their weight and to sum the weights.
- Use zip() and a list comprehension to iterate over the pairs of values and weights.
Sample Solution:
Python Code:
# Define a function 'weighted_average' that takes two lists 'nums' and 'weights'.
# The function calculates the weighted average by summing the product of corresponding elements in 'nums' and 'weights'
# and dividing it by the sum of 'weights'.
def weighted_average(nums, weights):
return sum(x * y for x, y in zip(nums, weights)) / sum(weights)
# Example 1: Create two lists 'nums1' and 'nums2' with elements.
nums1 = [10, 50, 40]
nums2 = [2, 5, 3]
print("Original list elements:")
print(nums1)
print(nums2)
# Call 'weighted_average' to compute the weighted average of 'nums1' and 'nums2'.
print("\nWeighted average of the said two lists of numbers:")
print(weighted_average(nums1, nums2))
# Example 2: Create two lists 'nums1' and 'nums2' with elements.
nums1 = [82, 90, 76, 83]
nums2 = [.2, .35, .45, 32]
print("\nOriginal list elements:")
print(nums1)
print(nums2)
# Call 'weighted_average' to compute the weighted average of 'nums1' and 'nums2'.
print("\nWeighted average of the said two lists of numbers:")
print(weighted_average(nums1, nums2))
Sample Output:
Original list elements: [10, 50, 40] [2, 5, 3] Weighted average of the said two list of numbers: 39.0 Original list elements: [82, 90, 76, 83] [0.2, 0.35, 0.45, 32] Weighted average of the said two list of numbers: 82.97272727272727
Flowchart:
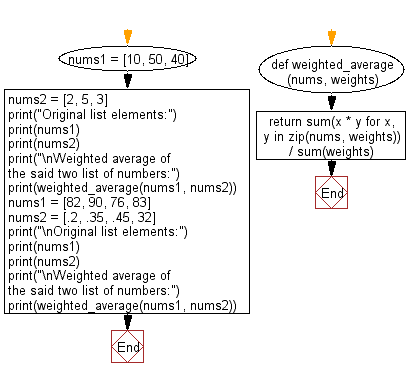
Python Code Editor:
Previous: Write a Python program to get the n minimum elements from a given list of numbers.
Next: Write a Python program to perform a deep flattens a list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics