Python: Check if two lists contain the same elements regardless of order
Compare Lists for Same Elements
Write a Python program to check if two given lists contain the same elements regardless of order.
- Use set() on the combination of both lists to find the unique values.
- Iterate over them with a for loop comparing the count() of each unique value in each list.
- Return False if the counts do not match for any element, True otherwise.
Sample Solution:
Python Code:
# Define a function to check if two lists contain the same elements regardless of order.
def check_same_contents(nums1, nums2):
# Loop through the set of elements in the combined lists.
for x in set(nums1 + nums2):
# Check if the count of element 'x' in nums1 is not equal to the count of 'x' in nums2.
if nums1.count(x) != nums2.count(x):
return False # If counts are not equal, the lists do not have the same contents.
return True # If all counts are equal for all elements, the lists have the same contents.
# Example 1
nums1 = [1, 2, 4]
nums2 = [2, 4, 1]
print("Original list elements:")
print(nums1)
print(nums2)
print("\nCheck two said lists contain the same elements regardless of order!")
print(check_same_contents(nums1, nums2))
# Example 2
nums1 = [1, 2, 3]
nums2 = [1, 2, 3]
print("\nOriginal list elements:")
print(nums1)
print(nums2)
print("\nCheck two said lists contain the same elements regardless of order!")
print(check_same_contents(nums1, nums2))
# Example 3
nums1 = [1, 2, 3]
nums2 = [1, 2, 4]
print("\nOriginal list elements:")
print(nums1)
print(nums2)
print("\nCheck two said lists contain the same elements regardless of order!")
print(check_same_contents(nums1, nums2))
Sample Output:
Original list elements: [1, 2, 4] [2, 4, 1] Check two said lists contain the same elements regardless of order! True Original list elements: [1, 2, 3] [1, 2, 3] Check two said lists contain the same elements regardless of order! True Original list elements: [1, 2, 3] [1, 2, 4] Check two said lists contain the same elements regardless of order! False
Flowchart:
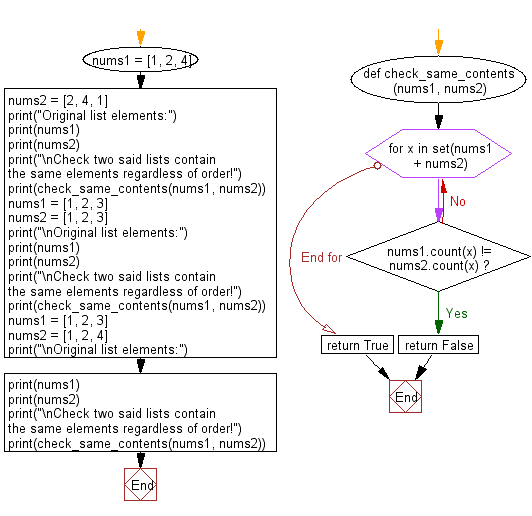
For more Practice: Solve these Related Problems:
- Write a Python program to check if two lists contain the same elements with identical frequencies, regardless of order.
- Write a Python program to compare two lists for set equality and then output the common elements and differences.
- Write a Python program to verify whether two lists contain the same unique elements after filtering out duplicates.
- Write a Python program to determine if two lists are permutations of each other by comparing sorted versions of both.
Python Code Editor:
Previous: Write a Python program to get the powerset of a given iterable.
Next: Write a Python program to create a given flat list of all the keys in a flat dictionary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics