Python: Create a given flat list of all the keys in a flat dictionary
Keys of Flat Dictionary
Write a Python program to create a flat list of all the keys in a flat dictionary.
- Use dict.keys() to return the keys in the given dictionary.
- Return a list() of the previous result.
Sample Solution:
Python Code:
# Define a function to extract and return a list of keys from a dictionary.
def keys_only(students):
return list(students.keys()) # Use the keys() method to get the keys from the dictionary.
# Create a dictionary of students with names as keys and their respective grades as values.
students = {
'Laura': 10,
'Spencer': 11,
'Bridget': 9,
'Howard ': 10, # Note: There is an extra space at the end of 'Howard'.
}
# Print the original dictionary elements.
print("Original directory elements:")
print(students)
# Call the 'keys_only' function to extract keys and print them as a flat list.
print("\nFlat list of all the keys of the said dictionary:")
print(keys_only(students))
Sample Output:
Original directory elements: {'Laura': 10, 'Spencer': 11, 'Bridget': 9, 'Howard ': 10} Flat list of all the keys of the said dictionary: ['Laura', 'Spencer', 'Bridget', 'Howard ']
Flowchart:
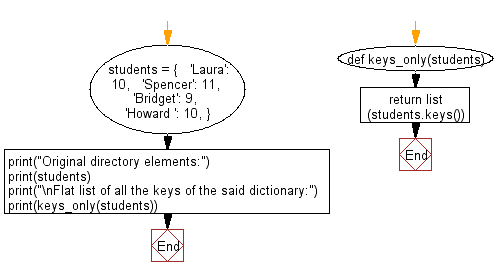
For more Practice: Solve these Related Problems:
- Write a Python program to create a flat list of keys from a nested dictionary by concatenating keys with a specified separator.
- Write a Python program to extract all keys from a flat dictionary and then sort them alphabetically.
- Write a Python program to generate a list of keys from a dictionary and filter out keys that do not match a given pattern.
- Write a Python program to retrieve keys from a dictionary and then compute the frequency of each key if duplicates exist.
Previous: Write a Python program to check if two given lists contain the same elements regardless of order.
Next: Write a Python program to check if a given function returns True for at least one element in the list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.